写一段将链表原地置逆的c语言代码
时间: 2023-07-25 20:41:08 浏览: 96
以下是将链表原地置逆的 C 语言代码:
```
// 定义链表结构体
struct ListNode {
int val;
struct ListNode *next;
};
struct ListNode* reverseList(struct ListNode* head) {
struct ListNode *prev = NULL;
struct ListNode *curr = head;
struct ListNode *next = NULL;
while (curr != NULL) {
next = curr->next;
curr->next = prev;
prev = curr;
curr = next;
}
return prev;
}
```
该函数接收一个链表头节点指针,通过遍历链表,依次将当前节点的 next 指针指向前一个节点,最后返回新的头节点指针,即原链表的尾节点指针。这样就完成了将链表原地置逆的操作。
相关问题
请写出一段链表插入结点的c语言代码
struct Node {
int data;
struct Node* next;
};
void insertNode(struct Node** head_ref, int new_data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
请为我写一段计算双向链表长度的c语言代码
以下是一个简单的计算双向链表长度的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* prev;
struct Node* next;
};
// 计算双向链表长度
int countNodes(struct Node* head) {
int count = 0;
struct Node* current = head;
while (current != NULL) {
count++;
current = current->next;
}
return count;
}
int main() {
// 创建双向链表
struct Node* head = (struct Node*)malloc(sizeof(struct Node));
struct Node* second = (struct Node*)malloc(sizeof(struct Node));
struct Node* third = (struct Node*)malloc(sizeof(struct Node));
head->data = 1;
head->prev = NULL;
head->next = second;
second->data = 2;
second->prev = head;
second->next = third;
third->data = 3;
third->prev = second;
third->next = NULL;
// 计算双向链表长度并打印结果
int length = countNodes(head);
printf("双向链表的长度为: %d\n", length);
// 释放内存
free(head);
free(second);
free(third);
return 0;
}
```
上述代码中,我们创建了一个双向链表,并定义了一个计算链表长度的函数`countNodes()`。函数中使用一个计数器`count`遍历整个链表,并在遍历每个节点时将计数器加1。最终返回计数器的值即为链表的长度。在主函数中,我们调用了`countNodes()`函数来计算链表长度,并打印结果。最后,我们释放了双向链表中的所有节点的内存。
阅读全文
相关推荐


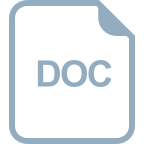













