把上述代码的注释改成中文
时间: 2023-05-29 08:03:32 浏览: 166
# 导入必要的库
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# 读取数据
data = pd.read_csv('data.csv')
# 将数据分为训练集和测试集
train_data = data[:80]
test_data = data[80:]
# 定义特征和目标变量
train_features = train_data[['x']]
train_labels = train_data['y']
test_features = test_data[['x']]
test_labels = test_data['y']
# 使用线性回归模型进行训练
from sklearn.linear_model import LinearRegression
model = LinearRegression()
model.fit(train_features, train_labels)
# 对测试集进行预测
predictions = model.predict(test_features)
# 将预测结果可视化
plt.scatter(test_features, test_labels, color='black')
plt.plot(test_features, predictions, color='blue', linewidth=3)
plt.xlabel('x')
plt.ylabel('y')
plt.show()
相关问题
能否将上述代码的注释改成中文
当然可以,以下是将上述代码中的英文注释翻译成中文:
```cpp
#include <iostream>
#include <pthread.h>
#include <unistd.h>
// 计算 f(x) 的函数
void* calculate_fx(void* arg) {
int x = *((int*)arg);
if (x == 1)
return &result_fx; // 如果 x 等于 1,则直接返回结果
result_fx = 1; // 初始化 f(x) 为 1
for (int i = 2; i <= x; ++i) { // 遍历从 2 到 x 的整数
result_fx *= i; // 按照定义更新 f(x)
write(fd_x, &result_fx, sizeof(result_fx)); // 将结果写入到 f(x) 的管道中
}
return NULL;
}
// 计算 f(y) 的函数
void* calculate_fy(void* arg) {
int y = *((int*)arg);
if (y <= 2)
return &result_fy; // 如果 y 小于等于 2,则直接返回结果
result_fy = 1; // 初始化 f(y) 为 1
for (int i = 2; i <= y; ++i) { // 遍历从 2 到 y 的整数
read(fd_y, &temp, sizeof(temp)); // 从 f(y) 的管道读取临时结果
result_fy += temp; // 根据读取的结果更新 f(y)
}
write(fd_y, &result_fy, sizeof(result_fy)); // 更新 f(y) 并将其写回管道
return NULL;
}
int main() {
// 创建用于 f(x) 和 f(y) 的管道
int fd_x[2], fd_y[2];
int ret;
pthread_t thread_fx, thread_fy;
// 对于 f(x),创建管道
if ((ret = pipe(fd_x)) != 0) {
std::cerr << "创建 f(x) 管道失败:" << perror("pipe") << std::endl;
return 1;
}
// 对于 f(y),同样创建管道
if ((ret = pipe(fd_y)) != 0) {
close(fd_x[0]);
close(fd_x[1]);
std::cerr << "创建 f(y) 管道失败:" << perror("pipe") << std::endl;
return 1;
}
// 启动线程,计算 f(x)
pthread_create(&thread_fx, NULL, calculate_fx, &x_value); // x_value 为要计算 f(x) 的值
// 启动线程,计算 f(y)
pthread_create(&thread_fy, NULL, calculate_fy, &y_value); // y_value 为要计算 f(y) 的值
// 等待两个线程结束
pthread_join(thread_fx, NULL);
pthread_join(thread_fy, NULL);
// 关闭管道
close(fd_x[0]);
close(fd_x[1]);
close(fd_y[0]);
close(fd_y[1]);
return 0;
}
```
这段代码中,我们首先包含了必要的头文件,然后定义了两个线程函数 `calculate_fx` 和 `calculate_fy`,这两个函数分别计算 `f(x)` 和 `f(y)` 的值。主线程创建了两个管道,分别用于传递 `f(x)` 和 `f(y)` 的计算结果,并启动相应的线程进行计算。当计算结束后,关闭了所有的管道。
请将上述注释改成中文
以下是中文注释的代码示例,演示如何使用CCS编写C语言程序来读取光电传感器的数据:
```
#include <msp430.h>
#include <stdio.h>
#include <stdlib.h>
#define ADC12_A_MEMORY ADC12MEM0
void init_adc(void);
int main(void)
{
WDTCTL = WDTPW | WDTHOLD; // 停用看门狗定时器
PM5CTL0 &= ~LOCKLPM5; // 禁用GPIO上电默认高阻抗模式,以激活先前配置的端口设置
init_adc(); // 初始化ADC
while (1)
{
ADC12CTL0 |= ADC12ENC | ADC12SC; // 启动转换
while (ADC12CTL1 & ADC12BUSY); // 等待转换完成
int adc_val = ADC12_A_MEMORY; // 读取ADC值
printf("ADC value: %d\n", adc_val);
}
}
void init_adc(void)
{
P1SEL1 |= BIT0; // 配置P1.0为模拟输入
P1SEL0 |= BIT0;
ADC12CTL0 = ADC12SHT0_2 | ADC12ON; // 打开ADC,设置采样保持时间
ADC12CTL1 = ADC12SHP; // 使用采样保持脉冲模式
ADC12CTL2 |= ADC12RES_2; // 设置12位分辨率
ADC12MCTL0 = ADC12INCH_0; // 选择A0作为输入通道
ADC12IER0 |= ADC12IE0; // 为通道0启用ADC中断
}
```
需要注意的是,具体的程序实现需要根据不同的硬件平台和传感器型号进行调整和修改。
阅读全文
相关推荐
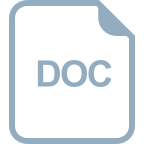
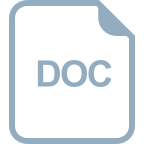
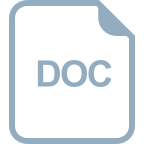

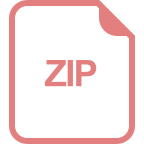
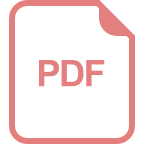
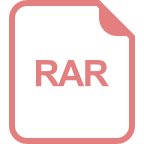





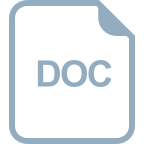
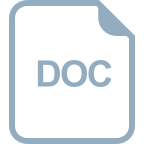
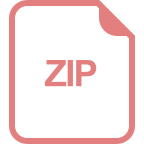
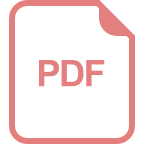