#include <iostream> #include <string> using namespace std; class Person{ public: void printInfo(){ cout << getInfo() << " "; } string getInfo(){ return "Person"; } }; class Student: public Person{ public: string getInfo(){ return "Student"; } }; int main(){ Person().printInfo(); Student().printInfo(); }解释输出
时间: 2024-03-02 14:54:17 浏览: 85
这段代码定义了一个 `Person` 类和 `Student` 类,其中 `Student` 类继承自 `Person` 类。在 `Person` 类中,定义了一个 `printInfo()` 方法和一个 `getInfo()` 方法,`printInfo()` 方法会调用 `getInfo()` 方法并输出返回值。在 `Student` 类中,也定义了一个 `getInfo()` 方法,该方法覆盖了基类 `Person` 中的同名方法。
在 `main()` 函数中,首先通过 `Person()` 创建了一个 `Person` 对象,然后调用了 `printInfo()` 方法。由于 `Person` 类中的 `getInfo()` 方法返回了 "Person",因此输出结果为 "Person"。
接下来,通过 `Student()` 创建了一个 `Student` 对象,然后同样调用了 `printInfo()` 方法。由于 `Student` 类中的 `getInfo()` 方法覆盖了 `Person` 类中的同名方法,因此输出结果为 "Student"。
因此,这段代码的输出结果为:
```
Person Student
```
相关问题
#include <iostream> #include <cmath>**** using namespace std; class Book { public: Book(const char *bookTitle) { pTitle = new char[strlen(bookTitle) + 1]; strcpy(pTitle, bookTitle); } void printInfo() const { cout << pTitle << endl; } ~Book() { delete pTitle;*** } private: char *pTitle; }; int main() { char bookTitle[60]; Book *pBook; cout << "Please enter book title: "; gets(bookTitle); Book book(); pBook = book; pBook.printInfo(); return 0; }
这段代码中有多处错误:
1. `#include <cmath>` 库未被使用,可以删除。
2. `using namespace std;` 没有错误。
3. 在类 `Book` 的析构函数中,应该使用 `delete[]` 释放 `new` 分配的数组内存,而不是使用 `delete`。
4. 在 `main` 函数中,应该使用 `std::cin` 和 `std::cout`,而不是 `gets` 和 `cout`。
5. 在 `main` 函数中,创建对象的方式不正确,应该使用 `new` 创建对象,并且调用对象的方法时应该使用箭头运算符 `->`。
下面是修改后的代码:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class Book {
public:
Book(const char *bookTitle) {
pTitle = new char[strlen(bookTitle) + 1];
strcpy(pTitle, bookTitle);
}
void printInfo() const {
cout << pTitle << endl;
}
~Book() {
delete[] pTitle;
}
private:
char *pTitle;
};
int main() {
char bookTitle[60];
Book *pBook;
cout << "Please enter book title: ";
cin.getline(bookTitle, 60);
pBook = new Book(bookTitle);
pBook->printInfo();
delete pBook;
return 0;
}
```
4 IntelliSense: 没有与这些操作数匹配的 "<<" 运算符 操作数类型为: std::ostream << std::string f:\cpp_program\Polymorphic_call\Polymorphic_call\polymorach.cpp 13 8 Polymorphic_call 但是显示这个报错,如何解决
这个报错是因为在代码中使用了cout,但是没有包含iostream头文件。请确保在代码中包含`#include <iostream>`这一行,以便引入iostream库并使用cout。修改后的代码应该如下所示:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Person {
public:
Person(const char* name): _name(name)
{
}
// 虚函数
virtual void BuyTicket()
{
cout << _name << "Person:买票-全价 100¥" << endl;
}
protected:
string _name;
// int _id;
};
```
这样修改后,应该能够成功编译和运行代码。
阅读全文
相关推荐











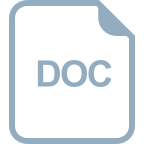


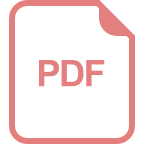
