vue项目three.js.Line顶点显示不同颜色
时间: 2024-03-17 17:42:48 浏览: 15
在Vue项目中使用`THREE.Line`来显示不同颜色的顶点,可以按照以下步骤进行:
1. 安装`three`库:在项目的根目录下运行以下命令安装`three`库。
```
npm install three
```
2. 在Vue组件中引入`three`库:在需要使用`THREE.Line`的Vue组件中引入`three`库。
```javascript
import * as THREE from 'three';
```
3. 定义顶点坐标和颜色数组:定义包含顶点坐标和颜色的数组,例如:
```javascript
const positions = [
0, 0, 0,
1, 1, 1,
2, 2, 2
];
const colors = [
1, 0, 0,
0, 1, 0,
0, 0, 1
];
```
4. 创建几何体和材质:使用`THREE.BufferGeometry`创建几何体,并将顶点坐标和颜色数组传递给几何体的`position`和`color`属性。然后,使用`THREE.LineBasicMaterial`创建材质,设置`vertexColors`为`THREE.VertexColors`。
```javascript
const geometry = new THREE.BufferGeometry();
geometry.addAttribute('position', new THREE.Float32BufferAttribute(positions, 3));
geometry.addAttribute('color', new THREE.Float32BufferAttribute(colors, 3));
const material = new THREE.LineBasicMaterial({ vertexColors: THREE.VertexColors });
```
5. 创建线对象并添加到场景中:使用几何体和材质创建`THREE.Line`对象,并将其添加到场景中。
```javascript
const line = new THREE.Line(geometry, material);
scene.add(line);
```
完整的Vue组件示例代码如下所示:
```vue
<template>
<div ref="container"></div>
</template>
<script>
import * as THREE from 'three';
export default {
mounted() {
// 创建场景、相机和渲染器
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
this.$refs.container.appendChild(renderer.domElement);
// 定义顶点坐标和颜色数组
const positions = [
0, 0, 0,
1, 1, 1,
2, 2, 2
];
const colors = [
1, 0, 0,
0, 1, 0,
0, 0, 1
];
// 创建几何体和材质
const geometry = new THREE.BufferGeometry();
geometry.addAttribute('position', new THREE.Float32BufferAttribute(positions, 3));
geometry.addAttribute('color', new THREE.Float32BufferAttribute(colors, 3));
const material = new THREE.LineBasicMaterial({ vertexColors: THREE.VertexColors });
// 创建线对象并添加到场景中
const line = new THREE.Line(geometry, material);
scene.add(line);
// 设置相机位置
camera.position.z = 5;
// 渲染场景
function animate() {
requestAnimationFrame(animate);
renderer.render(scene, camera);
}
animate();
}
}
</script>
<style>
#container {
width: 100%;
height: 100%;
overflow: hidden;
}
</style>
```
相关推荐
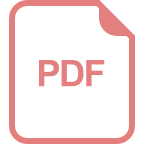














