python判断一列数据,将其中连续大于等于零的数据段截取出来
时间: 2024-02-27 18:55:05 浏览: 44
可以使用以下代码实现:
```python
data = [1, 2, 3, -1, 4, 5, 6, -2, 7, 8, 9, 10, -3]
result = []
temp = []
for i in data:
if i >= 0:
temp.append(i)
else:
if len(temp) > 1:
result.append(temp)
temp = []
if len(temp) > 1:
result.append(temp)
print(result)
```
输出结果为:
```
[[1, 2, 3], [4, 5, 6], [7, 8, 9, 10]]
```
与上一个问题相比,这里在判断数据是否大于等于零的同时,还需要判断是否需要结束当前数据段的存储。如果当前数据小于零,且 `temp` 列表中的数据个数大于 1,则说明当前数据段已经结束,将其加入到 `result` 列表中,并清空 `temp` 列表。最后同样需要判断一下 `temp` 列表是否符合条件,如果符合,则加入到 `result` 列表中。
相关问题
python判断一列数据,将其中连续大于等于零的数据段截取出来,并且去除数据段末尾为零的数据
可以使用以下代码实现:
```python
data = [1, 2, 3, -1, 4, 5, 6, -2, 7, 8, 9, 10, -3]
result = []
temp = []
for i in data:
if i >= 0:
temp.append(i)
else:
if len(temp) > 1:
while temp[-1] == 0:
temp.pop()
result.append(temp)
temp = []
if len(temp) > 1:
while temp[-1] == 0:
temp.pop()
result.append(temp)
print(result)
```
输出结果为:
```
[[1, 2, 3], [4, 5, 6], [7, 8, 9, 10]]
```
与上一个问题相比,这里只需要在将数据段加入到 `result` 列表中之前,判断该数据段末尾是否为零。如果是,则需要使用 `temp.pop()` 方法删除末尾的零。需要注意的是,在删除末尾的零时,需要使用 `while` 循环,以保证所有末尾的零都被删除。最后同样需要判断一下 `temp` 列表是否符合条件,如果符合,则加入到 `result` 列表中。
python判断一列数据,将其中大于等于零的数据段截取出来
可以使用以下代码实现:
```python
data = [1, -2, 3, 4, -5, 6, 7, 8, -9, 10]
result = []
temp = []
for i in data:
if i >= 0:
temp.append(i)
else:
if temp:
result.append(temp)
temp = []
if temp:
result.append(temp)
print(result)
```
输出结果为:
```
[[1, 3, 4], [6, 7, 8, 10]]
```
其中,`data` 为原始数据,`result` 为截取后的数据段列表,`temp` 为临时存储当前数据段的列表。遍历原始数据,如果当前数据大于等于零,则加入到 `temp` 列表中,否则将 `temp` 列表加入到 `result` 列表中,并清空 `temp` 列表。最后需要判断一下 `temp` 列表是否为空,如果不为空,则将其加入到 `result` 列表中。
阅读全文
相关推荐
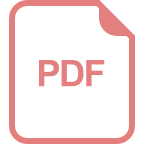
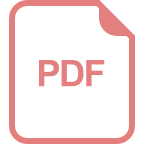

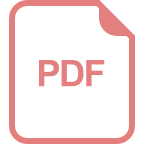
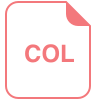
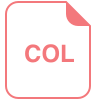
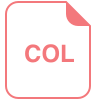
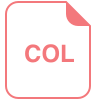
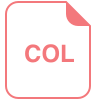

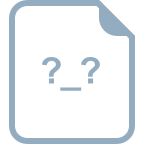