Python中 用七段数码管绘制HELLO的代码
时间: 2024-11-12 21:43:43 浏览: 8
在Python中,我们可以利用`pygame`库中的七段数码管模块来绘制文字,比如"HELLO"。首先,你需要安装`pygame`库,如果还没安装,可以使用`pip install pygame`命令。以下是一个简单的示例代码:
```python
import pygame
from pygame.locals import *
# 初始化Pygame
pygame.init()
# 设置窗口大小
display_width = 400
display_height = 200
screen = pygame.display.set_mode((display_width, display_height))
# 定义七段数码管字模
digit_dict = {
'0': ['### #', '### # #', '#### #', '#### #', '##### #', '##### #'],
'1': [' ### #', ' #### ', ' #####', ' # ', ' # ', ' # '],
'2': ['### # ', '#### #', '#### #', '#### #', '#### #', '### '],
... # 其他数字和字母的字模定义
}
def draw_char(char):
for digit in digit_dict[char]:
screen.fill((255, 255, 255), (10 + i * 60, 10, 50, 50)) # 绘制每个数码管的背景
for j, line in enumerate(digit.split()):
for pixel in line:
if pixel == '#':
pygame.draw.rect(screen, (0, 0, 0), (10 + i * 60 + j * 10, 10 + (5 - int(pixel)) * 10, 10, 10))
# 将字符串转换为对应的字符列表
message = "HELLO"
char_list = list(message)
# 主循环
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
quit()
screen.fill((255, 255, 255)) # 清屏
# 逐个绘制字符
for i, char in enumerate(char_list):
draw_char(char)
pygame.display.update() # 更新屏幕
```
这个代码会在一个小窗口中展示出"HELLO"的文字,由七段数码管的形式显示出来。注意实际应用中需要完整地定义所有的数字和字母字模,并且可能需要调整位置和其他细节。
阅读全文
相关推荐
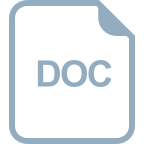
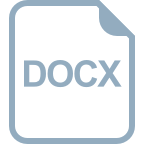
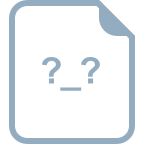


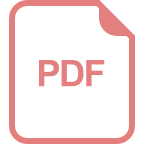
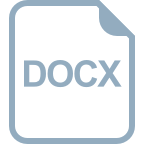
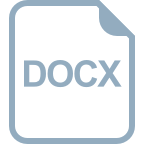
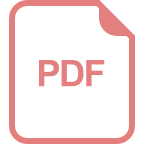
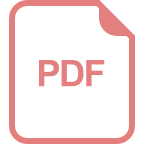
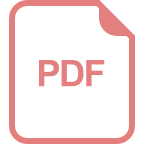
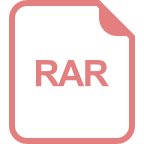
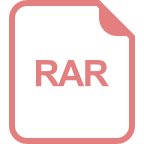
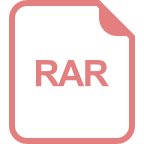
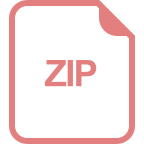
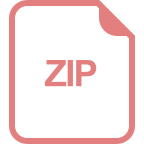