编写一个c语言程序,将秒转化为时钟标准格式
时间: 2024-10-25 16:05:30 浏览: 24
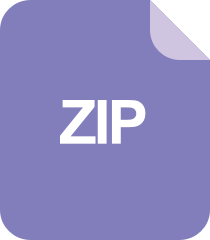
c语言电子时钟程序 zip
当然可以。在C语言中,我们可以编写一个函数来将秒数转换为小时、分钟和秒的标准时间格式。这里是一个简单的示例:
```c
#include <stdio.h>
#include <time.h>
// 函数来格式化秒数为HH:MM:SS
void convert_seconds_to_hms(long seconds) {
struct tm timeinfo;
time_t raw_time = seconds; // 将秒数转换成时间戳
localtime_r(&raw_time, &timeinfo); // 使用本地时间结构,获取当前时区的时间信息
int hours = timeinfo.tm_hour; // 小时
int minutes = timeinfo.tm_min; // 分钟
int seconds_left = timeinfo.tm_sec; // 秒
if (seconds_left >= 60) { // 如果有剩余秒超过60,需要进位到分钟
minutes++;
seconds_left %= 60;
}
if (minutes >= 60) { // 同理,如果分钟超过60,需要进位到小时
hours++;
minutes %= 60;
}
printf("%02d:%02d:%02d", hours, minutes, seconds_left);
}
int main() {
long seconds_input = 900; // 输入秒数作为示例
convert_seconds_to_hms(seconds_input);
return 0;
}
```
这个程序首先将输入的秒数转换为时间戳,然后使用`localtime_r`函数将其转换为本地时间结构。接着,通过处理`tm_info`中的小时、分钟和秒字段,并做必要的进位操作,最后将结果打印出来。
如果你想让用户输入秒数,只需在主函数中替换`seconds_input`即可。
阅读全文
相关推荐
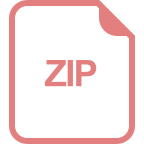
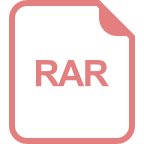
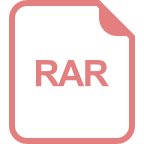
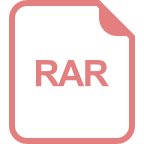
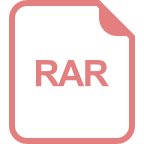
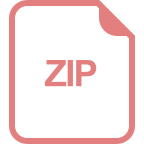
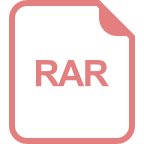
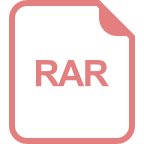
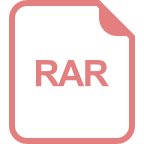
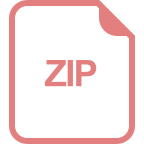
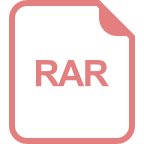
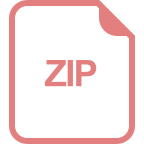
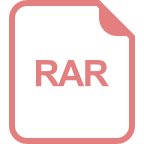
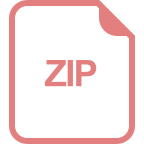
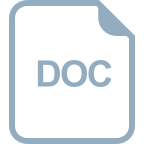
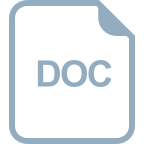
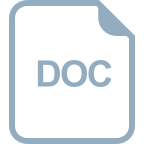
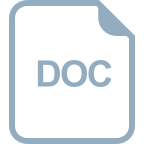