那我想将json文件的数据转存到现有的excel文件需要怎么写
时间: 2024-12-08 22:20:10 浏览: 4
如果你想要将JSON文件的数据转换并追加到现有的Excel文件中,你需要使用Python的`pandas`库,它提供了方便的数据操作功能,包括从JSON读取数据和与Excel交互。这里是一个简单的步骤示例:
首先,安装必要的库(如果尚未安装):
```bash
pip install pandas openpyxl
```
接下来,在Python代码中按照下面的步骤操作:
1. 导入所需的库:
```python
import json
import pandas as pd
from openpyxl import load_workbook
```
2. 加载JSON数据:
```python
def load_json_to_df(json_file_path):
with open(json_file_path, 'r') as file:
data = json.load(file)
df = pd.DataFrame(data)
return df
```
3. 加载并读取现有Excel文件,如果有需要,可以指定工作表名称:
```python
def append_excel(excel_file_path, new_data, sheet_name='Sheet1'):
# 加载现有Excel workbook
wb = load_workbook(excel_file_path)
# 获取或创建指定的工作表
ws = wb.get_sheet_by_name(sheet_name) if sheet_name else wb.active
# 将新数据追加到Excel中
ws.append(new_data)
# 保存修改后的workbook
wb.save(excel_file_path)
```
4. 调用这两个函数,将JSON数据加载到DataFrame,然后追加到Excel文件:
```python
json_file_path = "your_json_file.json"
excel_file_path = "your_excel_file.xlsx"
new_df = load_json_to_df(json_file_path)
append_excel(excel_file_path, new_df.to_list(), 'NewDataSheet') # 使用list形式追加,因为openpyxl接受二维列表
```
确保替换上述代码中的`your_json_file.json`和`your_excel_file.xlsx`为实际的文件路径。
阅读全文
相关推荐
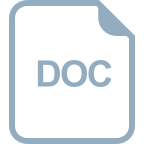
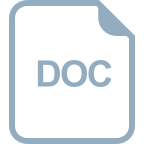
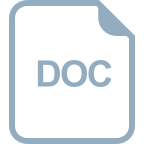
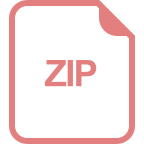
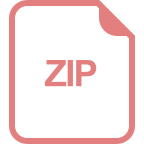
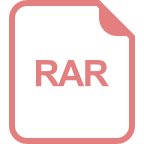
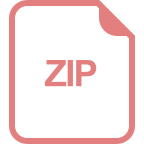
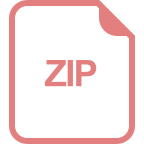
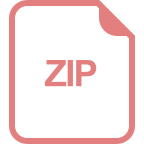
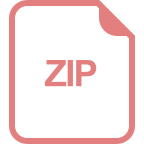
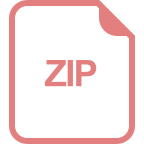
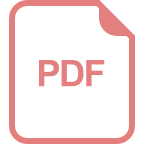
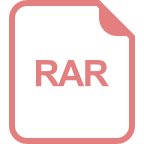
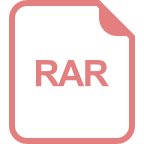
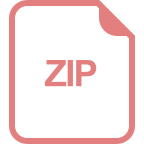
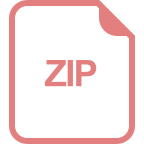