如何处理WPF DataGrid中的DataContextChanged事件?
时间: 2024-11-04 19:14:43 浏览: 22
在Windows Presentation Foundation (WPF)中的DataGrid控件中,`DataContextChanged`事件会在数据上下文(DataContext)更改时触发。这个事件通常用于更新UI以反映新的数据源。以下是处理这个事件的基本步骤:
1. 首先,你需要将DataGrid绑定到某个ObservableCollection或其他支持INotifyPropertyChanged的数据源上,这样当数据变化时,它会自动发送通知。
```xml
<DataGrid ItemsSource="{Binding MyDataSource}" />
```
2. 然后,在你的ViewModel或代码背后,需要添加对`DataContextChanged`事件的处理。这通常是在构造函数、依赖注入或者其他适当的地方:
```csharp
public class YourViewModel : INotifyPropertyChanged
{
private ObservableCollection<MyItem> _myDataSource;
public event PropertyChangedEventHandler PropertyChanged;
public YourViewModel()
{
_myDataSource = new ObservableCollection<MyItem>();
// 在这里注册 DataContext 变化事件
this.DataContextChanged += OnDataContextChanged;
}
private void OnDataContextChanged(object sender, DependencyPropertyChangedEventArgs e)
{
if (e.NewValue != null && e.NewValue is INotifyPropertyChanged)
{
// 当新的 DataContext 支持 INotifyPropertyChanged,更新DataGrid
((INotifyPropertyChanged)e.NewValue).PropertyChanged += (_, _) => OnPropertyChanged("MyDataSource");
}
else
{
// 如果新的 DataContext 不支持,清理之前的绑定了
((INotifyPropertyChanged)_myDataSource).PropertyChanged -= OnPropertyChanged;
}
// 更新DataGrid的内容
UpdateDataGrid();
}
protected virtual void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
private void UpdateDataGrid()
{
// 根据新的数据源刷新DataGrid内容
dataGrid.ItemsSource = _myDataSource;
}
}
```
在这个例子中,当DataGrid的数据上下文发生变化时,会检查新值是否实现了`INotifyPropertyChanged`。如果是,则订阅其`PropertyChanged`事件;如果不是,就取消之前的数据源绑定,并手动刷新DataGrid的内容。
阅读全文
相关推荐
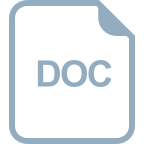
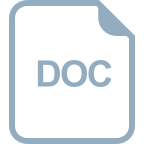
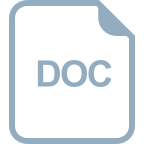
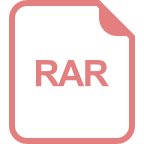
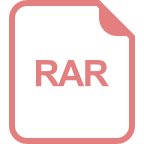
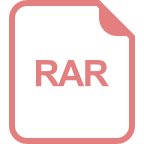
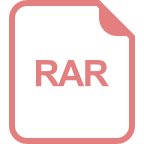
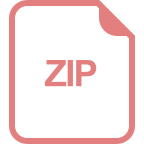
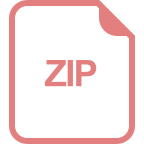
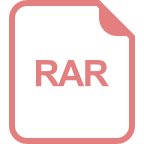
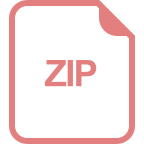
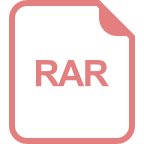
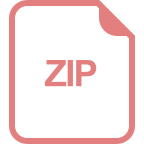
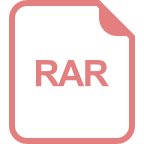
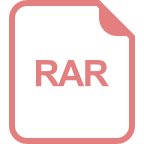
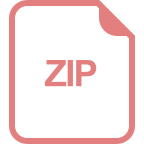
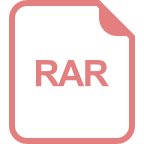
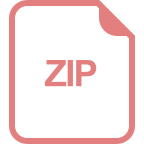
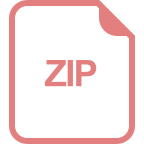