Blazor Services
时间: 2023-12-22 20:59:13 浏览: 30
Blazor Services are a feature in Blazor, a framework for building web applications using C# instead of JavaScript. Services in Blazor provide a way to share data and functionality across multiple components or pages in an application.
In Blazor, services can be registered and injected into components using dependency injection. This allows components to access and use the services without directly creating instances of the services themselves. Services can be used to encapsulate business logic, manage state, perform API calls, and more.
To create a service in Blazor, you would typically define an interface for the service, implement it in a class, and register it in the application's service container. Then, you can inject the service into your components or pages using the `@inject` directive or the `@inject` Razor directive.
Here's an example of creating and using a simple service in Blazor:
1. Define the service interface:
```csharp
public interface IMyService
{
string GetData();
}
```
2. Implement the service:
```csharp
public class MyService : IMyService
{
public string GetData()
{
return "Hello from MyService!";
}
}
```
3. Register the service in the application's service container:
```csharp
// In ConfigureServices method of Startup.cs
services.AddTransient<IMyService, MyService>();
```
4. Inject and use the service in a component:
```csharp
@inject IMyService myService
<p>@myService.GetData()</p>
```
In this example, the `IMyService` interface defines a method `GetData()`, which is implemented in the `MyService` class. The service is then registered with the `services` collection in the `ConfigureServices` method of the `Startup.cs` file. Finally, the service is injected into a component using the `@inject` directive and its method is called in the component's markup.
Blazor Services are a powerful tool for building modular and maintainable web applications with Blazor. They enable you to centralize and share functionality, making your code more organized and easier to test and maintain.
相关推荐
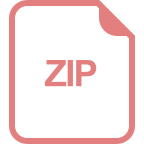
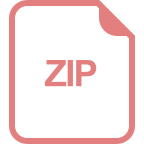
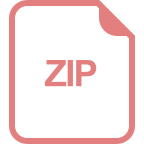














