编写一个基于SQLite3的通讯录管理系统。 本系统能实现添加记录、修改记录、删除记录、查询记录基本功能
时间: 2024-01-14 20:02:54 浏览: 24
好的,我可以为您提供一个Python实现的基于SQLite3的通讯录管理系统。
首先,我们需要安装Python的SQLite3模块,可以通过以下命令进行安装:
```
pip install pysqlite3
```
接下来,我们创建一个名为"contacts.db"的SQLite3数据库,并在其中创建一个名为"contacts"的表,该表包含以下字段:id, name, phone和email。
```
import sqlite3
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
c.execute('''CREATE TABLE contacts (id INTEGER PRIMARY KEY AUTOINCREMENT, name TEXT, phone TEXT, email TEXT)''')
conn.commit()
conn.close()
```
接下来,我们可以定义一些函数来实现添加记录、修改记录、删除记录和查询记录的基本功能。
```
import sqlite3
def add_contact(name, phone, email):
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
c.execute("INSERT INTO contacts (name, phone, email) VALUES (?, ?, ?)", (name, phone, email))
conn.commit()
conn.close()
def update_contact(id, name, phone, email):
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
c.execute("UPDATE contacts SET name = ?, phone = ?, email = ? WHERE id = ?", (name, phone, email, id))
conn.commit()
conn.close()
def delete_contact(id):
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
c.execute("DELETE FROM contacts WHERE id = ?", (id,))
conn.commit()
conn.close()
def search_contacts(name):
conn = sqlite3.connect('contacts.db')
c = conn.cursor()
c.execute("SELECT * FROM contacts WHERE name LIKE ?", ('%' + name + '%',))
contacts = c.fetchall()
conn.close()
return contacts
```
最后,我们可以编写一个简单的命令行界面来测试我们的通讯录管理系统。
```
while True:
print("1. Add Contact")
print("2. Update Contact")
print("3. Delete Contact")
print("4. Search Contacts")
print("5. Exit")
choice = input("Enter your choice: ")
if choice == '1':
name = input("Enter name: ")
phone = input("Enter phone: ")
email = input("Enter email: ")
add_contact(name, phone, email)
elif choice == '2':
id = input("Enter id: ")
name = input("Enter name: ")
phone = input("Enter phone: ")
email = input("Enter email: ")
update_contact(id, name, phone, email)
elif choice == '3':
id = input("Enter id: ")
delete_contact(id)
elif choice == '4':
name = input("Enter name: ")
contacts = search_contacts(name)
for contact in contacts:
print(contact)
elif choice == '5':
break
else:
print("Invalid choice. Please try again.")
```
这样,我们就完成了一个基于SQLite3的通讯录管理系统的编写。
相关推荐
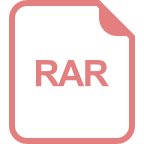
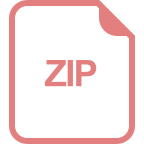
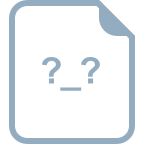







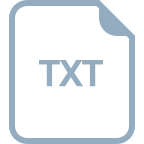
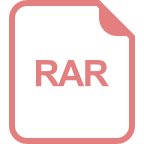
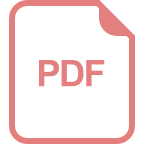
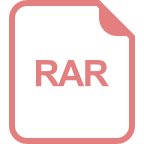
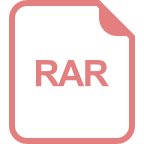
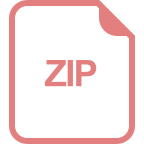
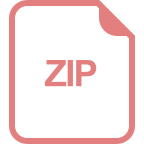
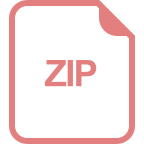