package org.example; public interface StudentDao { void list(); void delete(int id); } package org.example; public interface StudentService { void list(); void delete(int id); } package org.example; import org.springframework.stereotype.Repository; @Repository("mockStudentDao") public class MockStudentDao implements StudentDao{ @Override public void list() { System.out.println("All students are listed in MockStudentDao."); } @Override public void delete(int id) { System.out.println("Student No."+id+"is deleted in MockStudentDao."); } } package org.example; import org.springframework.stereotype.Repository; @Repository("myBatisStudentDao") public class MyBatisStudentDao implements StudentDao{ @Override public void list() { System.out.println("All students are listed in MyBatisStudentDao."); } @Override public void delete(int id) { System.out.println("Student No."+id+"is deleted in MyBatisStudentDao."); } } package org.example; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.stereotype.Service; @Service("studentServiceImpl") public class StudentServiceImpl implements StudentService{ private final StudentDao studentDao; @Autowired public StudentServiceImpl(@Qualifier("myBatisStudentDao")StudentDao studentDao){ this.studentDao=studentDao; } @Override public void list() { this.studentDao.list(); } @Override public void delete(int id) { this.studentDao.delete(id); } } package org.example; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class Main { public static void main(String[] args) { ApplicationContext ctx = new ClassPathXmlApplicationContext("beans.xml"); StudentService studentService = (StudentService) ctx.getBean("studentServiceImpl"); studentService.list(); studentService.delete(10); } }
时间: 2023-06-20 18:07:51 浏览: 52
这是一个使用 Spring 框架的 Java 代码示例,它实现了一个基本的三层架构,包括 DAO 层、Service 层和主函数入口。
其中,StudentDao 接口定义了两个方法:list() 和 delete(int id)。两个实现类 MockStudentDao 和 MyBatisStudentDao 分别实现了这两个方法。
StudentService 接口也定义了两个方法:list() 和 delete(int id)。StudentServiceImpl 实现了 StudentService 接口,并且它通过构造函数注入的方式,将 MyBatisStudentDao 对象注入到了 studentDao 属性中。
在 Main 类的 main 方法中,先通过 ClassPathXmlApplicationContext 加载了 beans.xml 配置文件,然后从中获取了 StudentService 对象,并且调用了它的 list() 和 delete(10) 方法。
这段代码是一个典型的基于 Spring 的三层架构的实现示例,它通过依赖注入的方式解耦了各个层之间的依赖关系,让代码更加灵活可维护。
相关问题
如果要使用@Resource注解实现装配,该如何修改代码package org.example; public interface StudentDao { void list(); void delete(int id); } package org.example; public interface StudentService { void list(); void delete(int id); } package org.example; import org.springframework.stereotype.Repository; @Repository("mockStudentDao") public class MockStudentDao implements StudentDao{ @Override public void list() { System.out.println("All students are listed in MockStudentDao."); } @Override public void delete(int id) { System.out.println("Student No."+id+"is deleted in MockStudentDao."); } } package org.example; import org.springframework.stereotype.Repository; @Repository("myBatisStudentDao") public class MyBatisStudentDao implements StudentDao{ @Override public void list() { System.out.println("All students are listed in MyBatisStudentDao."); } @Override public void delete(int id) { System.out.println("Student No."+id+"is deleted in MyBatisStudentDao."); } } package org.example; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.stereotype.Service; @Service("studentServiceImpl") public class StudentServiceImpl implements StudentService{ private final StudentDao studentDao; @Autowired public StudentServiceImpl(@Qualifier("myBatisStudentDao")StudentDao studentDao){ this.studentDao=studentDao; } @Override public void list() { this.studentDao.list(); } @Override public void delete(int id) { this.studentDao.delete(id); } } package org.example; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class Main { public static void main(String[] args) { ApplicationContext ctx = new ClassPathXmlApplicationContext("beans.xml"); StudentService studentService = (StudentService) ctx.getBean("studentServiceImpl"); studentService.list(); studentService.delete(10); } }如果要使用MockStudentDao
可以将StudentServiceImpl中的@Qualifier("myBatisStudentDao")修改为@Qualifier("mockStudentDao"),即:
@Service("studentServiceImpl")
public class StudentServiceImpl implements StudentService{
private final StudentDao studentDao;
@Autowired
public StudentServiceImpl(@Qualifier("mockStudentDao")StudentDao studentDao){
this.studentDao=studentDao;
}
@Override
public void list() {
this.studentDao.list();
}
@Override
public void delete(int id) {
this.studentDao.delete(id);
}
}
这样,Spring会将MockStudentDao注入到StudentServiceImpl中,从而实现装配。同时,在beans.xml中也需要将MockStudentDao的bean定义加入进去。
Dao: public interface StudentDao { void list(); void delete(int id); } @Repository public class MyBatisStudentDao implements StudentDao { @Override public void list() { System.out.println("MyBatisStudentDao 打印学生列表"); } @Override public void delete(int id) { System.out.println("MyBatisStudentDao 学生"+id+"已删除"); } } @Repository public class MockStudentDao implements StudentDao { @Override public void list() { System.out.println("MockStudentDao 打印学生列表"); } @Override public void delete(int id) { System.out.println("MockStudentDao 学生"+id+"已删除"); } } Service: public interface StudentService { void list(); void delete(int id); } @Service public class StudentServiceImpl implements StudentService { public final StudentDao studentDao; @Autowired public StudentServiceImpl(@Qualifier("myBatisStudentDao") StudentDao studentDao) { this.studentDao = studentDao; } @Override public void list() { studentDao.list(); } @Override public void delete(int id) { studentDao.delete(id); } } 测试类: public class main { @Test public void test(){ ApplicationContext ctx = new ClassPathXmlApplicationContext("applicationContext.xml"); StudentService studentService = (StudentService) ctx.getBean(StudentServiceImpl.class); studentService.list(); studentService.delete(2); } } 如果要使用@Resource注解实现装配,该如何修改代码
如果要使用@Resource注解实现装配,可以将StudentServiceImpl类中的@Autowired注解替换为@Resource注解,并指定对应的bean名称,例如:
```
@Service
public class StudentServiceImpl implements StudentService {
public final StudentDao studentDao;
@Resource(name="myBatisStudentDao")
public StudentServiceImpl(StudentDao studentDao) {
this.studentDao = studentDao;
}
@Override
public void list() {
studentDao.list();
}
@Override
public void delete(int id) {
studentDao.delete(id);
}
}
```
这样,Spring会自动从容器中查找名为"myBatisStudentDao"的bean,并将其注入到StudentServiceImpl对象中的studentDao属性中。在测试类中,可以保持不变,仍然使用ApplicationContext来加载配置文件并获取bean。
相关推荐






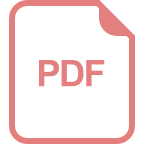
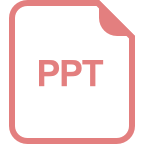
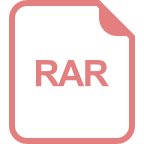
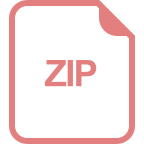