用idea,使用JFrame创建页面,能链接数据库的具有购物功能的代码
时间: 2024-02-29 20:57:27 浏览: 77
好的,这里是一个使用JFrame创建页面,能链接数据库的具有购物功能的Java代码示例:
```java
import javax.swing.*;
import java.awt.event.*;
import java.sql.*;
import java.util.Vector;
public class ShoppingSystem extends JFrame implements ActionListener {
private JLabel titleLabel = new JLabel("购物系统");
private JLabel nameLabel = new JLabel("商品名称:");
private JLabel priceLabel = new JLabel("商品价格:");
private JLabel quantityLabel = new JLabel("购买数量:");
private JTextField nameField = new JTextField();
private JTextField priceField = new JTextField();
private JTextField quantityField = new JTextField();
private JButton addButton = new JButton("添加到购物车");
private JButton checkoutButton = new JButton("结账");
private JTable cartTable = new JTable();
private Vector<String> columnNames = new Vector<String>();
private Vector<Vector<Object>> rowData = new Vector<Vector<Object>>();
private Connection conn;
public ShoppingSystem() {
// 设置窗口属性
setTitle("购物系统");
setSize(600, 400);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 添加组件
JPanel panel = new JPanel();
panel.setLayout(null);
panel.add(titleLabel);
panel.add(nameLabel);
panel.add(priceLabel);
panel.add(quantityLabel);
panel.add(nameField);
panel.add(priceField);
panel.add(quantityField);
panel.add(addButton);
panel.add(checkoutButton);
panel.add(new JScrollPane(cartTable));
// 设置组件位置和大小
titleLabel.setBounds(250, 20, 100, 30);
nameLabel.setBounds(50, 60, 80, 30);
nameField.setBounds(140, 60, 100, 30);
priceLabel.setBounds(50, 100, 80, 30);
priceField.setBounds(140, 100, 100, 30);
quantityLabel.setBounds(50, 140, 80, 30);
quantityField.setBounds(140, 140, 100, 30);
addButton.setBounds(50, 180, 100, 30);
checkoutButton.setBounds(160, 180, 80, 30);
cartTable.setBounds(300, 60, 250, 150);
// 设置表格列名
columnNames.add("商品名称");
columnNames.add("商品价格");
columnNames.add("购买数量");
// 添加按钮监听器
addButton.addActionListener(this);
checkoutButton.addActionListener(this);
// 连接数据库
try {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/shopping";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
// 显示窗口
setContentPane(panel);
setVisible(true);
}
public static void main(String[] args) {
new ShoppingSystem();
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
// 添加商品到购物车
String name = nameField.getText();
double price = Double.parseDouble(priceField.getText());
int quantity = Integer.parseInt(quantityField.getText());
Vector<Object> row = new Vector<Object>();
row.add(name);
row.add(price);
row.add(quantity);
rowData.add(row);
cartTable.setModel(new DefaultTableModel(rowData, columnNames));
} else if (e.getSource() == checkoutButton) {
// 结账
double totalPrice = 0;
for (int i = 0; i < rowData.size(); i++) {
String name = (String) rowData.get(i).get(0);
double price = (Double) rowData.get(i).get(1);
int quantity = (Integer) rowData.get(i).get(2);
totalPrice += price * quantity;
try {
String sql = "INSERT INTO orders (name, price, quantity) VALUES (?, ?, ?)";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, name);
pstmt.setDouble(2, price);
pstmt.setInt(3, quantity);
pstmt.executeUpdate();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
JOptionPane.showMessageDialog(this, "总价为:" + totalPrice);
}
}
}
```
说明:
1. 代码中使用了JFrame创建页面,包含了商品名称、商品价格、购买数量三个文本框和添加到购物车、结账两个按钮,以及一个表格用于显示购物车中的商品。
2. 代码中连接了MySQL数据库,使用了JDBC API。
3. 当点击添加到购物车按钮时,程序将会获取文本框中的数据,并将其添加到购物车表格中。
4. 当点击结账按钮时,程序将会遍历购物车表格中的商品,计算总价,并将订单信息插入到数据库中,最后弹出对话框显示总价。
阅读全文
相关推荐
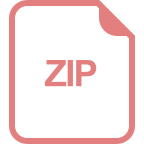

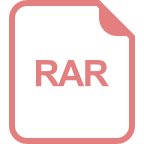
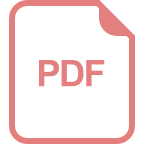
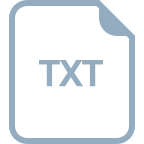
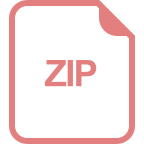
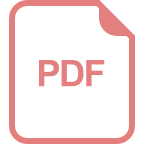
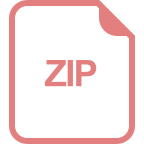
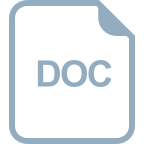
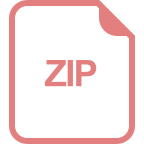
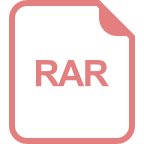
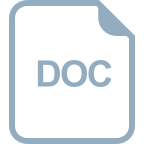

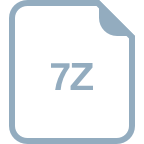
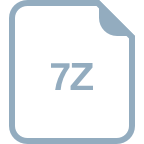
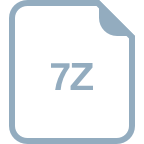