在Vue3中,如何详细地使用axios库来获取数据并将结果显示在页面上?
时间: 2024-12-22 09:17:04 浏览: 2
在 Vue3 中,使用 axios 库来获取数据并显示在页面上通常需要以下步骤:
1. 安装 axios:
首先,你需要安装 axios 作为 Vue 项目中的依赖。在命令行中运行:
```
npm install axios
```
或者如果你使用的是 yarn:
```
yarn add axios
```
2. 引入 Axios:
在你的 `.vue` 文件中或者在全局 `main.js` 文件中引入 axios:
```javascript
import axios from 'axios';
// 或者使用 ES6 模块导入
const axios = require('axios');
```
3. 创建实例(可选):
如果你想对 axios 设置全局配置,可以创建一个 axios 实例:
```javascript
const instance = axios.create({
baseURL: '你的 API 基础URL', // 如 https://api.example.com
headers: {'X-Custom-Header': 'foobar'}, // 自定义请求头
});
```
4. 在组件内发送请求:
在 Vue 组件的 methods 中编写一个方法用于发起 HTTP 请求:
```javascript
data() {
return {
loading: false,
response: null,
};
},
async fetchData() {
this.loading = true;
try {
const response = await instance.get('/your-endpoint'); // 替换为你实际的 API 路径
this.response = response.data; // 存储响应数据
} catch (error) {
console.error(error);
} finally {
this.loading = false; // 请求完成关闭加载状态
}
},
```
5. 显示数据:
在模板中绑定数据到视图:
```html
<template>
<div v-if="!loading">
<!-- 这里显示响应的数据 -->
{{ response }}
</div>
<button @click="fetchData">获取数据</button>
</template>
```
6. 触发请求:
现在你可以通过点击按钮或者其他触发事件的方式调用 `fetchData` 方法。
阅读全文
相关推荐
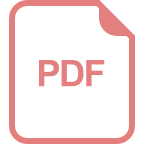
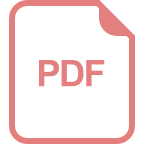
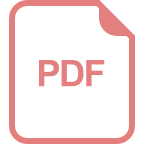
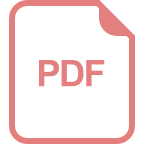
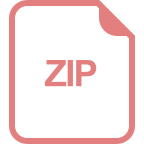
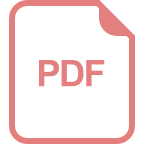
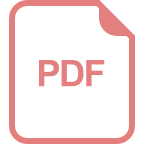
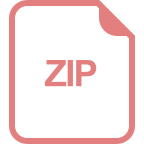
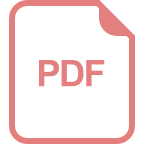
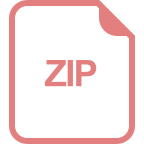
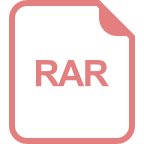
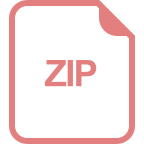
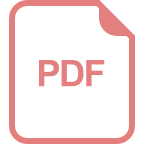
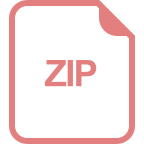
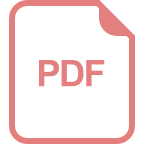
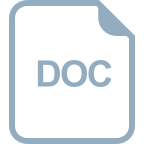
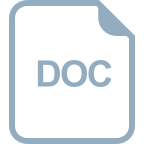
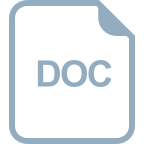
