统计指定字母开头单词的数量之用java
时间: 2023-08-31 22:42:23 浏览: 63
### 回答1:
可以使用Java中的HashMap来实现。
首先,读取文本文件中的单词,并将单词的首字母作为HashMap的键,值则为出现的次数。每读取一个单词,判断其首字母是否在HashMap中已经存在,如果存在则将该键对应的值加1,否则新建一个键值对。
最后,统计完成后遍历HashMap,输出每个字母开头的单词数量。
以下是示例代码:
```java
import java.util.*;
import java.io.*;
public class WordCounter {
public static void main(String[] args) throws IOException {
// 读取文本文件
BufferedReader reader = new BufferedReader(new FileReader("words.txt"));
// 创建HashMap
Map<Character, Integer> counterMap = new HashMap<>();
// 逐行读取单词
String line;
while ((line = reader.readLine()) != null) {
// 获取单词首字母
char firstChar = line.charAt(0);
// 判断首字母是否在HashMap中已经存在
if (counterMap.containsKey(firstChar)) {
// 如果存在,则将该键对应的值加1
counterMap.put(firstChar, counterMap.get(firstChar) + 1);
} else {
// 如果不存在,则新建一个键值对
counterMap.put(firstChar, 1);
}
}
// 关闭文件流
reader.close();
// 输出每个字母开头的单词数量
for (Map.Entry<Character, Integer> entry : counterMap.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
```
注意,以上代码中使用了try-with-resources语句来自动关闭文件流,需要Java 7及以上版本支持。如果是Java 6或更早的版本,可以使用try-catch-finally语句来手动关闭文件流。
### 回答2:
要统计指定字母开头的单词数量,可以使用Java编程语言来实现。
首先,我们需要一个存储单词的字符串数组或ArrayList。假设我们有一个名为words的字符串数组。
接下来,我们可以使用一个计数器变量来记录以指定字母开头的单词数量,初始化为0。
然后,我们可以使用forEach循环遍历字符串数组中的每个单词。
在循环内部,我们可以使用String类的charAt方法获取单词的首字母,并将其与指定字母进行比较。如果相等,说明该单词以指定字母开头,我们将计数器变量加1。
循环结束后,计数器变量的值就代表了以指定字母开头的单词数量。
下面是实现的代码示例:
```
public class WordCounter {
public static void main(String[] args) {
String[] words = {"apple", "banana", "cat", "dog", "elephant"};
char targetLetter = 'a';
int count = 0;
for (String word : words) {
if (word.charAt(0) == targetLetter) {
count++;
}
}
System.out.println("以字母 " + targetLetter + " 开头的单词数量为: " + count);
}
}
```
在上述示例代码中,我们假设目标字母是'a',字符串数组包含了一些单词。通过遍历每个单词,并比较其首字母与目标字母是否相等,计算出以指定字母开头的单词数量,并打印输出结果。
希望这个回答能帮助到你!
### 回答3:
要统计指定字母开头的单词数量,可以使用Java编程语言。下面是一个简单的实现例子:
```java
import java.util.Scanner;
public class WordCount {
public static void main(String[] args) {
// 创建一个Scanner对象,用于接收用户输入
Scanner scanner = new Scanner(System.in);
// 提示用户输入一段文字
System.out.println("请输入一段文字:");
String text = scanner.nextLine();
// 提示用户输入指定字母
System.out.println("请输入指定字母:");
char targetLetter = scanner.nextLine().charAt(0);
// 将输入的文字分割成单词
String[] words = text.split("[^a-zA-Z]+");
// 统计以指定字母开头的单词数量
int count = 0;
for (String word : words) {
if (word.toLowerCase().startsWith(String.valueOf(targetLetter).toLowerCase())) {
count++;
}
}
// 打印结果
System.out.println("以字母 " + targetLetter + " 开头的单词数量为:" + count);
}
}
```
以上代码通过使用Scanner类获取用户输入的文字和指定字母,并使用String的split方法将文字分割成单词。接着,通过遍历每个单词,并使用startsWith方法检查单词是否以指定字母开头,从而统计指定字母开头的单词数量。最后,打印结果给用户。
相关推荐
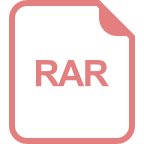
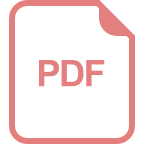















