利用哈希表来实现LRU页面置换算法C语言
时间: 2023-08-15 12:43:11 浏览: 55
好的,下面是一个利用哈希表和双向链表实现LRU页面置换算法的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define CACHE_CAPACITY 5 // 缓存容量为5
// 定义缓存节点
typedef struct CacheNode {
int key; // 缓存键
int value; // 缓存值
struct CacheNode *prev; // 上一个节点指针
struct CacheNode *next; // 下一个节点指针
} CacheNode;
// 定义哈希表节点
typedef struct HashNode {
int key; // 哈希键
CacheNode *value; // 哈希值,为指向缓存节点的指针
struct HashNode *next; // 下一个节点指针
} HashNode;
// 初始化缓存节点
CacheNode *initCacheNode(int key, int value) {
CacheNode *node = (CacheNode *)malloc(sizeof(CacheNode));
node->key = key;
node->value = value;
node->prev = NULL;
node->next = NULL;
return node;
}
// 初始化哈希表
HashNode **initHashTable() {
HashNode **hashTable = (HashNode **)malloc(CACHE_CAPACITY * sizeof(HashNode *));
for (int i = 0; i < CACHE_CAPACITY; i++) {
hashTable[i] = (HashNode *)malloc(sizeof(HashNode));
hashTable[i]->key = -1; // 哈希键设为-1,表示无效节点
hashTable[i]->value = NULL;
hashTable[i]->next = NULL;
}
return hashTable;
}
// 计算哈希值
int hash(int key) {
return key % CACHE_CAPACITY;
}
// 向哈希表中添加节点
void addHashNode(HashNode **hashTable, int key, CacheNode *node) {
int index = hash(key);
HashNode *hashNode = hashTable[index];
while (hashNode->next != NULL) { // 找到哈希表尾部
hashNode = hashNode->next;
}
HashNode *newNode = (HashNode *)malloc(sizeof(HashNode)); // 创建新节点
newNode->key = key;
newNode->value = node;
newNode->next = NULL;
hashNode->next = newNode; // 将新节点插入哈希表尾部
}
// 从哈希表中删除节点
void removeHashNode(HashNode **hashTable, int key) {
int index = hash(key);
HashNode *hashNode = hashTable[index];
while (hashNode->next != NULL) {
if (hashNode->next->key == key) { // 找到待删除节点
HashNode *temp = hashNode->next;
hashNode->next = temp->next; // 将待删除节点从哈希表中移除
free(temp);
break;
}
hashNode = hashNode->next;
}
}
// 查找哈希表中是否存在指定键
CacheNode *findHashNode(HashNode **hashTable, int key) {
int index = hash(key);
HashNode *hashNode = hashTable[index];
while (hashNode->next != NULL) {
if (hashNode->next->key == key) { // 找到指定键的哈希节点
return hashNode->next->value;
}
hashNode = hashNode->next;
}
return NULL;
}
// 将缓存节点移动到链表头部
void moveToHead(CacheNode *node) {
CacheNode *prev = node->prev;
CacheNode *next = node->next;
prev->next = next;
next->prev = prev;
node->prev = NULL;
node->next = NULL;
prev = next = node;
}
// 利用LRU算法获取缓存中指定键的值
int getCacheValue(HashNode **hashTable, CacheNode **head, CacheNode **tail, int key, int value) {
CacheNode *node = findHashNode(hashTable, key);
if (node != NULL) { // 如果键已经在缓存中,将其移动到链表头部
moveToHead(node);
return node->value;
} else { // 如果不在缓存中,将其添加到链表头部
node = initCacheNode(key, value);
addHashNode(hashTable, key, node);
node->next = *head;
(*head)->prev = node;
*head = node;
if (*tail == NULL) { // 如果链表为空,将尾指针指向头指针
*tail = *head;
}
if (hashTable[key % CACHE_CAPACITY]->next->next != NULL) { // 如果缓存已经满了,将链表尾部的节点移除
HashNode *lastNode = hashTable[(*tail)->key % CACHE_CAPACITY];
removeHashNode(hashTable, (*tail)->key);
*tail = (*tail)->prev;
(*tail)->next = NULL;
free(lastNode->next);
}
return -1;
}
}
int main() {
HashNode **hashTable = initHashTable();
CacheNode *head = NULL;
CacheNode *tail = NULL;
int cache[][2] = {{1, 10}, {2, 20}, {3, 30}, {4, 40}, {5, 50}, {6, 60}, {7, 70}, {8, 80}, {9, 90}, {1, 100}, {2, 200}, {3, 300}, {4, 400}}; // 测试数据
for (int i = 0; i < sizeof(cache) / sizeof(cache[0]); i++) {
int value = getCacheValue(hashTable, &head, &tail, cache[i][0], cache[i][1]);
if (value != -1) {
printf("hit: %d\n", value);
} else {
printf("miss: %d\n", cache[i][1]);
}
}
return 0;
}
```
以上就是一个基于哈希表和双向链表实现的LRU页面置换算法的C语言代码示例。
相关推荐
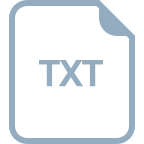
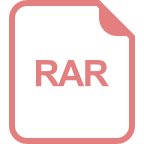














