给我一个用opencv python,利用realsense相机,实现识别“白色立方体”的程序。color画面经过高斯模糊、中值滤波等实现颜色的识别。然后结合depth深度数据,排除干扰
时间: 2023-05-28 11:03:46 浏览: 287
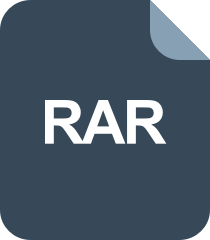
白色大气风格的建筑商业网站模板下载.rar
的物体,最终定位出白色立方体的位置。
以下是一个简单的示例代码,用于识别白色立方体:
```
import cv2
import numpy as np
import pyrealsense2 as rs
# 初始化realsense相机
pipeline = rs.pipeline()
config = rs.config()
config.enable_stream(rs.stream.color, 640, 480, rs.format.bgr8, 30)
config.enable_stream(rs.stream.depth, 640, 480, rs.format.z16, 30)
pipeline.start(config)
# 定义白色的颜色范围
white_lower = np.array([0, 0, 200], dtype=np.uint8)
white_upper = np.array([255, 30, 255], dtype=np.uint8)
while True:
# 获取相机图像
frames = pipeline.wait_for_frames()
color_frame = frames.get_color_frame()
depth_frame = frames.get_depth_frame()
if not color_frame or not depth_frame:
continue
# 将color图像转换为numpy数组
color_image = np.asanyarray(color_frame.get_data())
# 高斯模糊和中值滤波
blurred = cv2.GaussianBlur(color_image, (11, 11), 0)
median = cv2.medianBlur(blurred, 5)
# 将图像从BGR转换为HSV
hsv = cv2.cvtColor(median, cv2.COLOR_BGR2HSV)
# 根据设定的颜色范围进行颜色识别,并生成二值图像
mask = cv2.inRange(hsv, white_lower, white_upper)
mask = cv2.erode(mask, None, iterations=2)
mask = cv2.dilate(mask, None, iterations=2)
# 找到白色区域的轮廓
contours, hierarchy = cv2.findContours(mask.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
if len(contours) > 0:
# 找到最大的轮廓,并计算其外接矩形
c = max(contours, key=cv2.contourArea)
rect = cv2.minAreaRect(c)
box = cv2.boxPoints(rect)
box = np.int0(box)
# 计算白色立方体的中心点
center_x = int(rect[0][0])
center_y = int(rect[0][1])
# 获取白色立方体的深度值
depth = depth_frame.get_distance(center_x, center_y)
# 显示图像和深度值
cv2.drawContours(color_image, [box], 0, (0, 255, 0), 2)
cv2.putText(color_image, "Depth: {:.2f}m".format(depth), (30, 30), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 2)
else:
# 如果没有找到白色立方体,则清空深度值
depth = 0
# 显示图像
cv2.imshow("Color Image", color_image)
cv2.imshow("Mask", mask)
key = cv2.waitKey(1)
if key == ord('q'):
break
# 关闭所有窗口
cv2.destroyAllWindows()
pipeline.stop()
```
阅读全文
相关推荐
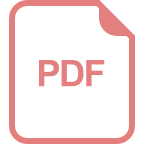
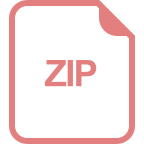
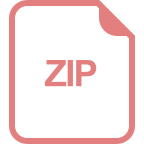
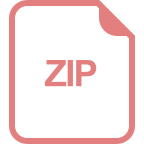
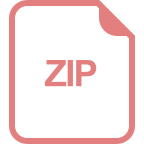