使用java语言运用swing创建一个景点详细信息界面,使用表格结构,包含编号,景点名称,景点位置,联系电话,价格,景点介绍,照片框,其中景点位置框中除文字外包含旅游导览图链接,页面最后包含点赞,收藏,评论,返回按钮
时间: 2024-04-28 09:26:38 浏览: 28
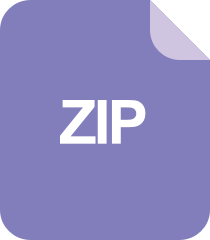
毕设 用java写的swing界面,也用javasocket写的通讯,各种功能都用java实现
下面是一个简单的 Java Swing 界面实现,包含了您所需的表格结构和各个组件。您可以根据具体需求进行调整。
```java
import javax.swing.*;
import javax.swing.table.DefaultTableModel;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ScenicSpotDetailFrame extends JFrame {
private JLabel idLabel, nameLabel, locationLabel, phoneLabel, priceLabel, introductionLabel, photoLabel;
private JTextField idField, nameField, locationField, phoneField, priceField;
private JTextArea introductionArea;
private JButton likeButton, collectButton, commentButton, backButton;
private JTable photoTable;
private JScrollPane photoScrollPane;
public ScenicSpotDetailFrame() {
setTitle("景点详细信息");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(800, 600);
// 创建表格模型
DefaultTableModel photoTableModel = new DefaultTableModel(new Object[][]{}, new Object[]{"照片"});
photoTable = new JTable(photoTableModel);
photoScrollPane = new JScrollPane(photoTable);
// 创建标签和文本框
idLabel = new JLabel("编号:");
nameLabel = new JLabel("景点名称:");
locationLabel = new JLabel("景点位置:");
phoneLabel = new JLabel("联系电话:");
priceLabel = new JLabel("价格:");
introductionLabel = new JLabel("景点介绍:");
photoLabel = new JLabel("照片:");
idField = new JTextField();
nameField = new JTextField();
locationField = new JTextField();
phoneField = new JTextField();
priceField = new JTextField();
introductionArea = new JTextArea();
// 创建点赞、收藏、评论和返回按钮
likeButton = new JButton("点赞");
collectButton = new JButton("收藏");
commentButton = new JButton("评论");
backButton = new JButton("返回");
// 创建面板
JPanel infoPanel = new JPanel(new GridLayout(7, 2));
JPanel buttonPanel = new JPanel(new FlowLayout());
JPanel photoPanel = new JPanel(new BorderLayout());
// 设置面板布局
infoPanel.add(idLabel);
infoPanel.add(idField);
infoPanel.add(nameLabel);
infoPanel.add(nameField);
infoPanel.add(locationLabel);
infoPanel.add(locationField);
infoPanel.add(phoneLabel);
infoPanel.add(phoneField);
infoPanel.add(priceLabel);
infoPanel.add(priceField);
infoPanel.add(introductionLabel);
infoPanel.add(introductionArea);
infoPanel.add(photoLabel);
infoPanel.add(photoScrollPane);
buttonPanel.add(likeButton);
buttonPanel.add(collectButton);
buttonPanel.add(commentButton);
buttonPanel.add(backButton);
photoPanel.add(photoScrollPane, BorderLayout.CENTER);
// 添加面板到窗口
add(infoPanel, BorderLayout.NORTH);
add(buttonPanel, BorderLayout.SOUTH);
add(photoPanel, BorderLayout.CENTER);
}
public static void main(String[] args) {
ScenicSpotDetailFrame frame = new ScenicSpotDetailFrame();
frame.setVisible(true);
}
}
```
在这个示例中,我们使用了多个 Swing 组件来实现界面:
- JLabel 和 JTextField :用于显示标签和文本框,用于显示编号、景点名称、景点位置、联系电话、价格等信息。
- JTextArea :用于显示景点介绍。
- JTable :用于显示照片列表。
- JScrollPane :用于包含照片列表,使其可以滚动。
- JButton :用于创建点赞、收藏、评论和返回按钮。
- JPanel :用于创建面板,用于布局各个组件。
您可以在每个按钮的事件处理程序中添加具体的功能,例如点赞、收藏、评论等。
阅读全文
相关推荐
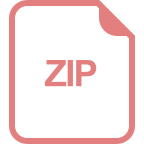
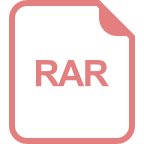
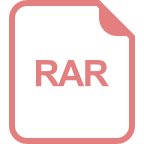
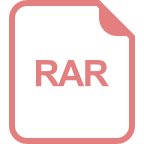
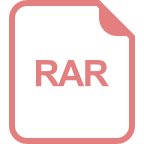
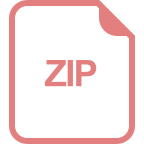
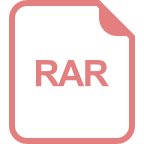
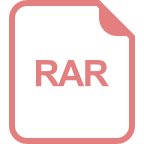
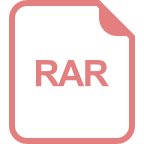
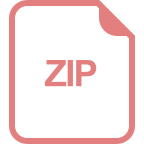
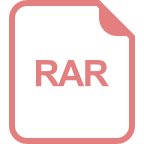
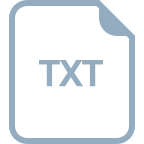
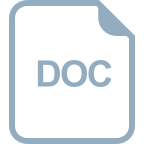
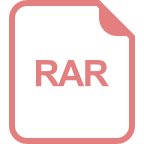
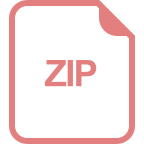
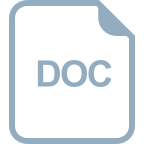