Python中如何创建更复杂形状的爱心代码?
时间: 2024-10-07 14:02:17 浏览: 21
在Python中,你可以通过各种库如`turtle`来绘制复杂的图形,包括爱心。`turtle`是一个内置的绘图模块,非常适合教学和初学者学习基本的图形操作。下面是一个简单的例子,展示如何使用`turtle`画出一个比较复杂的爱心:
```python
import turtle
# 创建一个Turtle对象并设置颜色
tess = turtle.Turtle()
tess.color('red')
# 定义函数绘制爱心的一部分
def draw_half_heart(length):
tess.forward(length)
tess.left(140) # 向左转140度
tess.forward(length * 0.707) # 直线长度的一半用于形成心尖
tess.right(60)
tess.forward(length)
tess.left(50)
tess.forward(length)
# 绘制完整的爱心,两次调整方向
draw_half_heart(100)
tess.right(180) # 转向相反方向
draw_half_heart(100)
# 关闭窗口
turtle.done()
相关问题
如何在Python中自定义更复杂的爱心图案?
在Python中,自定义更复杂的爱心图案可以通过迭代和条件判断来实现。除了基本的心形符号,还可以结合其他形状、线条或者其他ASCII字符来创造个性化的样式。以下是一个示例,创建了一个稍微复杂一点的爱心形状:
```python
def custom_heart(width=10):
# 上半部分
top_half = [' ' * int((width - 2) / 2), '*' * width, ' ' * int((width - 2) / 2)]
# 下半部分
bottom_half = [
' ' * int((width - 2) / 2),
'V' * ((width - 4) // 2) + '^\n' + 'V' * ((width - 4) // 2),
' ' * int((width - 2) / 2)
]
return '\n'.join(top_half + bottom_half)
print(custom_heart())
```
这个函数可以根据宽度参数调整爱心的大小。运行这个代码将显示一个包含星号(*)和字符'V'的心形图案。
Python爱心代码可以用哪些方法创建更复杂的形状?
Python爱心代码通常是非常基础的,如果你想创建更复杂的形状,可以考虑扩展到多行爱心或者结合其他字符。例如,你可以尝试添加更多的层级、旋转爱心,甚至将多个爱心组合成图案。这里有一个简单例子,展示如何添加层次和变化:
```python
def heart_pattern(n, orientation='vertical'):
for i in range(n):
if orientation == 'vertical':
print(' ' * (n - 1) + '*' * (i+1) + ' ' * ((n-i-1)*2) + ' V ' * (i+1) + '*' * (n-i-1))
else:
print(' ' * (n - 1) + ' H ' * (i+1) + ' ' * ((n-i-1)*2) + '*' * (i+1) + ' ' * (n-1))
heart_pattern(5, 'horizontal') # 可以试试不同的方向和规模
```
这个代码会打印出带有"V"和"H"的垂直和水平爱心,增加了多样性。
阅读全文
相关推荐
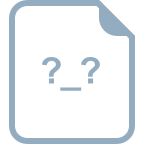
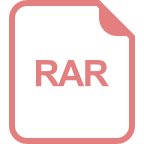
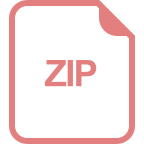













