用c++编程判断一个英文句子有多少个单词
时间: 2024-12-06 14:24:11 浏览: 27
在C++中,判断一个英文句子中有多少个单词,可以采用分隔字符串并计数的方式来实现。一种常见的方法是利用空格、标点符号作为分隔符。下面是一个简单的示例:
```cpp
#include <iostream>
#include <sstream>
#include <string>
int countWords(const std::string& sentence) {
std::istringstream iss(sentence); // 创建输入流
std::string word;
int count = 0;
while (iss >> word) { // 逐词读取,直到无法获取更多单词
++count; // 每次读取到单词就加一
}
return count; // 返回单词总数
}
int main() {
std::string input_sentence = "Hello, how are you today?";
int word_count = countWords(input_sentence);
std::cout << "The sentence has " << word_count << " words.\n";
return 0;
}
```
这个程序首先将输入的句子转换为输入流(istringstream),然后在一个循环里尝试从流中读取单词,每读取一次就增加计数器。当流无法再读取到单词时(即已达到句尾),停止循环。
相关问题
在c++输入一个字符串,编程统计其单词个数及字母个数
好的,下面是代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str;
int wordCount = 0, letterCount = 0;
cout << "请输入一个字符串:" << endl;
getline(cin, str);
for (int i = 0; i < str.length(); i++) {
// 判断是否为字母
if (isalpha(str[i])) {
letterCount++;
// 判断是否为单词的结尾
if (i == str.length() - 1 || str[i + 1] == ' ') {
wordCount++;
}
}
}
cout << "单词个数:" << wordCount << endl;
cout << "字母个数:" << letterCount << endl;
return 0;
}
```
这里使用了 `isalpha()` 函数判断是否为字母,使用了 `getline()` 函数输入一行字符串。遍历字符串时,如果遇到字母,就增加字母计数器,同时判断是否为单词的结尾,如果是,则增加单词计数器。最后输出单词个数和字母个数。
C++输入一行字符串(字符串长度 ≤100 ),包含若干个单词,约定相邻的两个单词用空格隔开(一个或多个空格),编程统计单词的个数。
您可以使用以下代码来统计单词的个数:
```cpp
#include <iostream>
#include <string>
using namespace std;
int countWords(string str) {
int count = 0;
bool isWord = false;
// 遍历字符串的每个字符
for (char c : str) {
// 判断当前字符是否是字母或数字
if (isalnum(c)) {
// 如果当前字符是字母或数字,并且之前不是单词,则将单词计数增加
if (!isWord) {
count++;
isWord = true;
}
}
else {
// 如果当前字符不是字母或数字,则之后的字符不属于单词
isWord = false;
}
}
return count;
}
int main() {
string str;
cout << "请输入一行字符串: ";
getline(cin, str);
int wordCount = countWords(str);
cout << "单词的个数为: " << wordCount << endl;
return 0;
}
```
这段代码会提示用户输入一行字符串,并通过 `getline()` 函数获取输入的字符串。然后,`countWords()` 函数会遍历字符串的每个字符,判断是否是字母或数字,从而统计出单词的个数。最后,程序会输出单词的个数。
请注意,这段代码假设单词只包含字母和数字,如果您的需求中还包含其他字符,请根据实际情况进行修改。
阅读全文
相关推荐
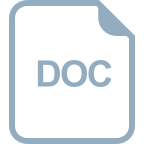
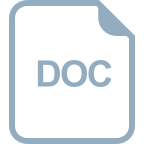
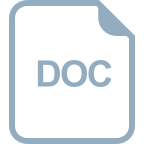
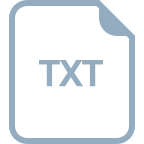
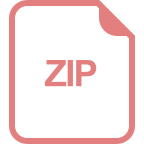
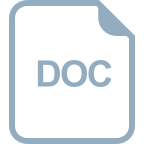
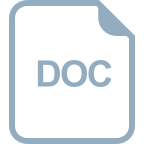
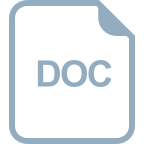
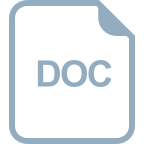
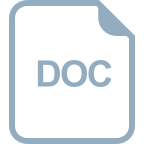
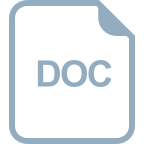
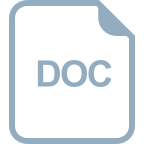
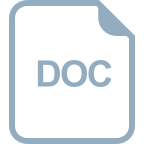
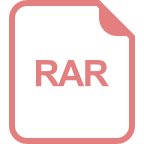
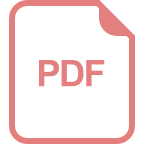
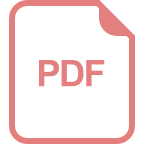