class Wardrobe { private ArrayList<clothes> clothesList = new ArrayList<>(); //添加的函数 public void addClothes(clothes Clothes) { //提供了添加方法 //该方法创建了衣物 clothesList.add(Clothes); } //删除的函数 public void removeClothes(int index) { //提供了删除方法 clothesList.remove(index); } //查看的函数 public void listClothes() { //添加默认衣服 Scanner scanner=new Scanner(System.in); clothes newClothes = new clothes("默认衣物", "黑色", 38); addClothes(newClothes); //提供了查看方法 //通过进行for循环来查看多件衣服衣服 //for循环的实现 for (int i = 0; i < clothesList.size(); i++) { //获取衣服的属性 clothes clothes =clothesList.get(i); //输出衣服 System.out.println((i + 1) + ". " + clothes.getName() + " (" + clothes.getColor() + ", " + clothes.getSize() ); } } //添加方法可以修改衣物信息 public void updateClothes(int index,String name,String color,int size) { //对衣物进行实现 clothes clothes=clothesList.get(index); //衣物的名字 clothes.name=name; //衣物的颜色 clothes.color=color; //衣物的尺寸 clothes.size=size; clothesList.set(index,clothes); System.out.println("衣物的信息修改成功"); } //添加方法可以清空衣柜 public void clearClothes() { clothesList.clear(); System.out.println("衣柜已清空!"); } 修改代码
时间: 2024-02-23 09:02:00 浏览: 19
好的,您想修改搜索方法的代码,那么我先帮您补充一下缺失的搜索方法。您可以参考下面的代码实现:
```java
//搜索的函数
public void searchClothes(String keyword) {
//通过进行for循环来搜索衣物
//for循环的实现
for (int i = 0; i < clothesList.size(); i++) {
//获取衣服的属性
clothes clothes = clothesList.get(i);
//判断衣物名字是否包含关键字
if (clothes.getName().contains(keyword)) {
//输出匹配的衣物信息
System.out.println((i + 1) + ". " + clothes.getName() + " (" + clothes.getColor() + ", " + clothes.getSize() );
}
}
}
```
搜索方法的实现原理是通过循环遍历衣柜中的每件衣物,判断衣物名字是否包含关键字,如果包含则输出匹配的衣物信息。您可以在调用该方法时传入关键字,例如 `wardrobe.searchClothes("T恤")`,即可搜索名字中包含 "T恤" 的衣物信息。
相关问题
class Wardrobe { private ArrayList<clothes> clothesList = new ArrayList<>(); //添加的函数 public void addClothes(clothes Clothes) { //提供了添加方法 //该方法创建了衣物 clothesList.add(Clothes); } //删除的函数 public void removeClothes(int index) { //提供了删除方法 clothesList.remove(index); } //查看的函数 public void listClothes() { //添加默认衣服 Scanner scanner=new Scanner(System.in); clothes newClothes = new clothes("默认衣物", "黑色", 38); addClothes(newClothes); //无法录入衣物怎么修改
在你的代码中,已经提供了添加衣物的方法,但是在查看函数中添加默认衣物的代码不应该放在这个位置。你需要将添加默认衣物的代码放在Wardrobe类的构造函数中,这样每次创建一个新的Wardrobe对象时都会自动添加这个默认衣物。修改后的代码如下:
```
class Wardrobe {
private ArrayList<clothes> clothesList = new ArrayList<>();
public Wardrobe() {
// 添加默认衣物
clothes newClothes = new clothes("默认衣物", "黑色", 38);
addClothes(newClothes);
}
// 添加的函数
public void addClothes(clothes Clothes) {
clothesList.add(Clothes);
}
// 删除的函数
public void removeClothes(int index) {
clothesList.remove(index);
}
// 查看的函数
public void listClothes() {
System.out.println("衣柜中的衣物列表:");
for (clothes c : clothesList) {
System.out.println(c);
}
}
}
```
这样,每次创建一个Wardrobe对象时,都会自动添加一个默认衣物,并且可以使用listClothes()方法来查看衣柜中的所有衣物。
class Wardrobe { private ArrayList<clothes> clothesList = new ArrayList<>(); //添加的函数 public void addClothes(clothes Clothes) { //提供了添加方法 //该方法创建了衣物 clothesList.add(Clothes); } //删除的函数 public void removeClothes(int index) { //提供了删除方法 clothesList.remove(index); } //查看的函数 public void listClothes() { //添加默认衣服 Scanner scanner=new Scanner(System.in); //提供了查看方法 //通过进行for循环来查看多件衣服衣服 //for循环的实现 for (int i = 0; i < clothesList.size(); i++) { //获取衣服的属性 clothes clothes =clothesList.get(i); //输出衣服 System.out.println((i + 1) + ". " + clothes.getName() + " (" + clothes.getColor() + ", " + clothes.getSize() ); } }为什么不能录入衣物怎么修改
要添加一个函数或方法来让用户录入衣物的属性,您可以添加一个名为addNewClothes()的函数,该函数接收用户的输入并创建一个新的clothes对象,最后将其添加到clothesList列表中。以下是一个可能的修改版本:
```
import java.util.Scanner;
public class Wardrobe {
private ArrayList<clothes> clothesList = new ArrayList<>();
public void addClothes(clothes Clothes) {
clothesList.add(Clothes);
}
public void removeClothes(int index) {
clothesList.remove(index);
}
public void listClothes() {
for (int i = 0; i < clothesList.size(); i++) {
clothes clothes = clothesList.get(i);
System.out.println((i + 1) + ". " + clothes.getName() + " (" + clothes.getColor() + ", " + clothes.getSize() + ")");
}
}
public void addNewClothes() {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the name of the clothes: ");
String name = scanner.nextLine();
System.out.print("Enter the color of the clothes: ");
String color = scanner.nextLine();
System.out.print("Enter the size of the clothes: ");
String size = scanner.nextLine();
clothes newClothes = new clothes(name, color, size);
addClothes(newClothes);
}
}
```
现在,您可以在需要添加新衣物时调用addNewClothes()函数。
相关推荐
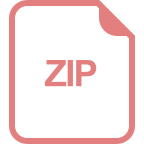
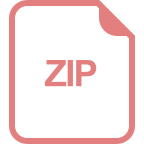
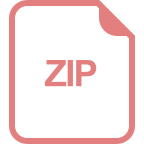



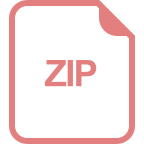
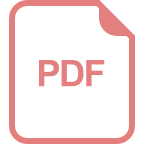
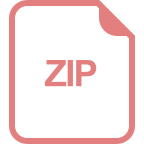
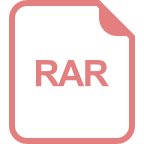
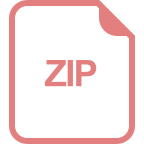