在C# Winform .NET4.8中,每秒绘制5000个动态数据点显示瀑布图使用OxyPlot库,并根据数据点大小自动调整颜色?能否提供一个包含数据生成和颜色映射的完整示例代码?
时间: 2024-10-22 21:12:34 浏览: 56
在C# WinForms .NET4.8中使用OxyPlot库绘制每秒5000个动态数据点的瀑布图,并根据数据点的大小自动调整颜色,可以通过以下步骤实现:
1. 安装OxyPlot库。
2. 创建WinForms应用程序。
3. 使用OxyPlot控件来显示图表。
4. 生成动态数据并更新图表。
5. 根据数据点大小调整颜色。
以下是一个完整的示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows.Forms;
using OxyPlot;
using OxyPlot.Series;
using OxyPlot.Axes;
using OxyPlot.WindowsForms;
namespace WaterfallChartExample
{
public partial class Form1 : Form
{
private PlotView plotView;
private LineSeries lineSeries;
private Random random = new Random();
private List<double> dataPoints = new List<double>();
private Timer timer;
public Form1()
{
InitializeComponent();
InitializePlot();
StartDataGeneration();
}
private void InitializePlot()
{
plotView = new PlotView
{
Dock = DockStyle.Fill,
Location = new System.Drawing.Point(0, 0),
Name = "plotView",
Size = new System.Drawing.Size(800, 600),
TabIndex = 0,
Text = "Waterfall Chart"
};
this.Controls.Add(plotView);
var model = new PlotModel { Title = "Dynamic Waterfall Chart" };
lineSeries = new LineSeries { MarkerType = MarkerType.Circle };
model.Series.Add(lineSeries);
plotView.Model = model;
}
private void StartDataGeneration()
{
timer = new Timer();
timer.Interval = 1000 / 5000; // Generate 5000 points per second
timer.Tick += OnTimerTick;
timer.Start();
}
private void OnTimerTick(object sender, EventArgs e)
{
double newValue = random.NextDouble() * 100; // Generate a random value between 0 and 100
dataPoints.Add(newValue);
UpdateChart();
}
private void UpdateChart()
{
lineSeries.Points.Clear();
for (int i = 0; i < dataPoints.Count; i++)
{
double y = dataPoints[i];
OxyColor color = GetColorForValue(y);
lineSeries.Points.Add(new DataPoint(i, y) { Color = color });
}
plotView.InvalidatePlot(true);
}
private OxyColor GetColorForValue(double value)
{
// Map value to a color range from blue (low) to red (high)
double normalizedValue = Math.Min(Math.Max(value / 100, 0), 1);
return OxyColor.FromRgb((byte)(normalizedValue * 255), 0, (byte)((1 - normalizedValue) * 255));
}
}
}
```
### 说明:
1. **初始化PlotView和LineSeries**:在`InitializePlot`方法中,我们创建了一个`PlotView`和一个`LineSeries`,并将它们添加到表单中。
2. **启动数据生成**:在`StartDataGeneration`方法中,我们设置了一个定时器,每秒钟生成5000个数据点。
3. **更新图表**:在`OnTimerTick`事件处理程序中,我们生成一个新的随机值并将其添加到数据列表中,然后调用`UpdateChart`方法更新图表。
4. **根据数据点大小调整颜色**:在`GetColorForValue`方法中,我们将数据点的值映射到蓝色到红色的渐变色。
这个示例展示了如何使用OxyPlot库在WinForms应用程序中实时绘制瀑布图,并根据数据点的大小自动调整颜色。你可以根据需要进一步优化和扩展此示例。
阅读全文
相关推荐





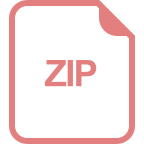


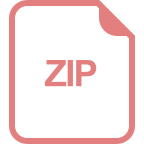



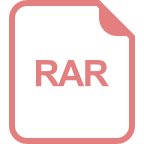
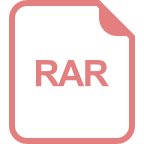


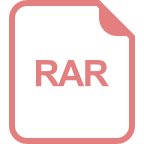

