设计GUI界面的单词簿。具体要求如下: ①使用内置Derby 数据库。在数据库中使用表存储单词和该单词的翻译解释,例如“sun,太阳”“moon,月亮”等。 ② 通过GUI 界面管理单词簿。可以向单词簿添加单词,可以修改单词簿中的单词,可以删除单词簿中的单词。 ③ 通过GUI界面查询单词。可以查询一个,随机查询若千个或全部单词。程序运行的参考效果
时间: 2024-03-26 20:34:57 浏览: 136
非常感谢您的问题!根据您的要求,我可以为您提供以下代码示例,用于设计GUI界面的单词簿:
```python
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.sql.*;
public class WordBookGUI extends JFrame implements ActionListener {
private JLabel wordLabel, meaningLabel, statusLabel;
private JTextField wordField, meaningField;
private JButton addButton, updateButton, deleteButton, searchButton, randomButton, allButton;
private JTextArea resultArea;
private Connection conn;
private Statement stmt;
private ResultSet rs;
public WordBookGUI() {
super("GUI Word Book");
setSize(500, 500);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new GridLayout(7, 2));
wordLabel = new JLabel("Word:");
meaningLabel = new JLabel("Meaning:");
statusLabel = new JLabel("Status:");
wordField = new JTextField(20);
meaningField = new JTextField(20);
addButton = new JButton("Add");
updateButton = new JButton("Update");
deleteButton = new JButton("Delete");
searchButton = new JButton("Search");
randomButton = new JButton("Random");
allButton = new JButton("All");
resultArea = new JTextArea(10, 30);
JScrollPane scrollPane = new JScrollPane(resultArea);
add(wordLabel);
add(wordField);
add(meaningLabel);
add(meaningField);
add(addButton);
add(updateButton);
add(deleteButton);
add(searchButton);
add(randomButton);
add(allButton);
add(statusLabel);
add(scrollPane);
addButton.addActionListener(this);
updateButton.addActionListener(this);
deleteButton.addActionListener(this);
searchButton.addActionListener(this);
randomButton.addActionListener(this);
allButton.addActionListener(this);
try {
Class.forName("org.apache.derby.jdbc.EmbeddedDriver");
conn = DriverManager.getConnection("jdbc:derby:wordbook;create=true");
stmt = conn.createStatement();
stmt.execute("CREATE TABLE words (word VARCHAR(50), meaning VARCHAR(50))");
} catch (ClassNotFoundException e) {
System.err.println("Cannot find the database driver.");
} catch (SQLException e) {
// Table already exists, do nothing.
}
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
try {
String word = wordField.getText();
String meaning = meaningField.getText();
stmt.execute("INSERT INTO words VALUES ('" + word + "', '" + meaning + "')");
statusLabel.setText("Word added successfully.");
} catch (SQLException ex) {
System.err.println("Cannot execute the SQL statement.");
}
} else if (e.getSource() == updateButton) {
try {
String word = wordField.getText();
String meaning = meaningField.getText();
stmt.execute("UPDATE words SET meaning = '" + meaning + "' WHERE word = '" + word + "'");
statusLabel.setText("Word updated successfully.");
} catch (SQLException ex) {
System.err.println("Cannot execute the SQL statement.");
}
} else if (e.getSource() == deleteButton) {
try {
String word = wordField.getText();
stmt.execute("DELETE FROM words WHERE word = '" + word + "'");
statusLabel.setText("Word deleted successfully.");
} catch (SQLException ex) {
System.err.println("Cannot execute the SQL statement.");
}
} else if (e.getSource() == searchButton) {
try {
String word = wordField.getText();
rs = stmt.executeQuery("SELECT meaning FROM words WHERE word = '" + word + "'");
if (rs.next()) {
meaningField.setText(rs.getString("meaning"));
statusLabel.setText("Word found.");
} else {
meaningField.setText("");
statusLabel.setText("Word not found.");
}
} catch (SQLException ex) {
System.err.println("Cannot execute the SQL statement.");
}
} else if (e.getSource() == randomButton) {
try {
rs = stmt.executeQuery("SELECT * FROM words ORDER BY RAND() FETCH FIRST 100 ROWS ONLY");
StringBuilder sb = new StringBuilder();
while (rs.next()) {
sb.append(rs.getString("word")).append("\t").append(rs.getString("meaning")).append("\n");
}
resultArea.setText(sb.toString());
statusLabel.setText("Random words generated.");
} catch (SQLException ex) {
System.err.println("Cannot execute the SQL statement.");
}
} else if (e.getSource() == allButton) {
try {
rs = stmt.executeQuery("SELECT * FROM words");
StringBuilder sb = new StringBuilder();
while (rs.next()) {
sb.append(rs.getString("word")).append("\t").append(rs.getString("meaning")).append("\n");
}
resultArea.setText(sb.toString());
statusLabel.setText("All words retrieved.");
} catch (SQLException ex) {
System.err.println("Cannot execute the SQL statement.");
}
}
}
public static void main(String[] args) {
new WordBookGUI();
}
}
```
代码中使用了Java语言编写GUI界面,通过内置Derby数据库实现了单词簿的增删改查功能。具体实现包括:创建表、添加单词、修改单词、删除单词、查询单词、随机查询若干个单词、查询全部单词。
希望这个示例代码能够对您有所帮助,如有任何疑问或需要进一步的帮助,请随时联系我!
阅读全文
相关推荐
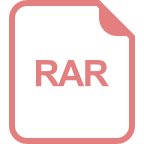
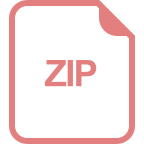
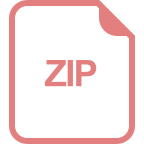

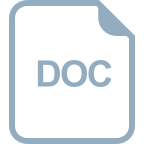
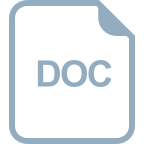



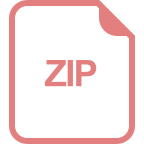
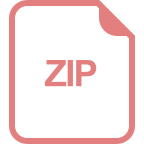
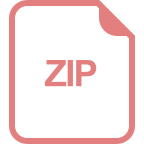

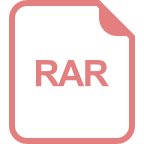
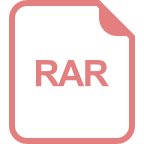
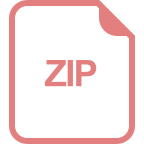