使用Swing提供的组件创建一个类似金山打字通的程序,显示键盘按键,统计用户每分钟输入的字符数目、正确字符数目、正确率。
时间: 2023-06-26 14:10:33 浏览: 44
以下是一个简单的示例代码,实现了一个类似金山打字通的程序:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.border.*;
public class TypingProgram extends JFrame implements ActionListener {
private JLabel lblKey;
private JTextField txtInput;
private JTextArea txtResult;
private int totalCharCount, correctCharCount, incorrectCharCount;
private long startTime;
public TypingProgram() {
super("Typing Program");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(640, 480);
setResizable(false);
JPanel panel = new JPanel(new GridLayout(4, 1));
panel.setBorder(new EmptyBorder(10, 10, 10, 10));
lblKey = new JLabel("Press Any Key to Start Typing");
lblKey.setFont(new Font("Arial", Font.BOLD, 24));
lblKey.setHorizontalAlignment(JLabel.CENTER);
panel.add(lblKey);
txtInput = new JTextField();
txtInput.setFont(new Font("Arial", Font.PLAIN, 18));
txtInput.setHorizontalAlignment(JTextField.CENTER);
txtInput.addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e) {
if (startTime == 0) {
startTime = System.currentTimeMillis();
new Thread(new TimerThread()).start();
}
char key = e.getKeyChar();
lblKey.setText(String.valueOf(key));
if (key == txtResult.getText().charAt(0)) {
correctCharCount++;
txtResult.setText(txtResult.getText().substring(1));
if (txtResult.getText().length() == 0) {
showResult();
}
} else {
incorrectCharCount++;
}
totalCharCount++;
}
});
panel.add(txtInput);
txtResult = new JTextArea();
txtResult.setEditable(false);
txtResult.setFont(new Font("Arial", Font.PLAIN, 18));
txtResult.setLineWrap(true);
txtResult.setWrapStyleWord(true);
txtResult.setText("The quick brown fox jumps over the lazy dog.");
panel.add(txtResult);
getContentPane().add(panel);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equals("Restart")) {
totalCharCount = 0;
correctCharCount = 0;
incorrectCharCount = 0;
startTime = 0;
lblKey.setText("Press Any Key to Start Typing");
txtInput.setText("");
txtResult.setText("The quick brown fox jumps over the lazy dog.");
}
}
private void showResult() {
long totalTime = System.currentTimeMillis() - startTime;
double accuracy = ((double) correctCharCount / totalCharCount) * 100;
double speed = ((double) totalCharCount / totalTime) * 1000 * 60;
String result = String.format("Time: %.2f seconds, Speed: %.2f cpm, Accuracy: %.2f%%",
totalTime / 1000.0, speed, accuracy);
JOptionPane.showMessageDialog(this, result, "Result", JOptionPane.INFORMATION_MESSAGE);
txtInput.setText("");
txtResult.setText("The quick brown fox jumps over the lazy dog.");
lblKey.setText("Press Any Key to Start Typing");
totalCharCount = 0;
correctCharCount = 0;
incorrectCharCount = 0;
startTime = 0;
}
private class TimerThread implements Runnable {
public void run() {
try {
Thread.sleep(5000);
showResult();
} catch (InterruptedException e) {
}
}
}
public static void main(String[] args) {
new TypingProgram();
}
}
```
在这个程序中,我们使用了 Swing 提供的 JLabel、JTextField、JTextArea 和 JOptionPane 组件,分别用于显示键盘按键、接受用户输入、显示文本和显示统计结果。
在键盘输入时,我们使用了 KeyListener 接口来监听用户输入的按键,并根据用户输入的字符来判断输入是否正确。同时,我们还使用了 TimerThread 类来实现一个计时器,计算用户输入的速度和准确率,并在一定时间后弹出结果对话框。
注意,由于本程序只是一个简单的示例,所以在实际使用中可能需要进一步完善。例如,可以增加更多的练习文本、统计更多的数据、支持多种练习模式等等。
相关推荐
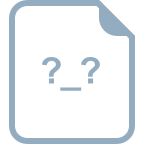
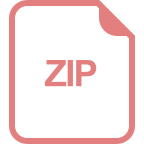














