对孩子兄弟链表的树计算树的深度,用c++编写
时间: 2023-03-19 15:21:39 浏览: 172
孩子兄弟链表的树可以使用递归的方式计算树的深度。若树为空,则深度为0;否则,树的深度等于其子树中深度最大值加1。
以下是使用C语言编写的孩子兄弟链表树深度计算的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 树节点结构体
typedef struct TreeNode {
int data;
struct TreeNode *firstChild;
struct TreeNode *nextSibling;
} TreeNode;
// 创建一个新节点
TreeNode* createNode(int data) {
TreeNode *newNode = (TreeNode*)malloc(sizeof(TreeNode));
newNode->data = data;
newNode->firstChild = NULL;
newNode->nextSibling = NULL;
return newNode;
}
// 插入子节点
void insertChild(TreeNode *parent, TreeNode *child) {
if (parent == NULL || child == NULL) {
return;
}
if (parent->firstChild == NULL) {
parent->firstChild = child;
} else {
TreeNode *sibling = parent->firstChild;
while (sibling->nextSibling != NULL) {
sibling = sibling->nextSibling;
}
sibling->nextSibling = child;
}
}
// 计算树的深度
int calculateDepth(TreeNode *root) {
if (root == NULL) {
return 0;
}
int maxDepth = 0;
TreeNode *child = root->firstChild;
while (child != NULL) {
int childDepth = calculateDepth(child);
if (childDepth > maxDepth) {
maxDepth = childDepth;
}
child = child->nextSibling;
}
return maxDepth + 1;
}
int main() {
// 创建一个孩子兄弟链表的树
TreeNode *root = createNode(1);
TreeNode *node2 = createNode(2);
TreeNode *node3 = createNode(3);
TreeNode *node4 = createNode(4);
TreeNode *node5 = createNode(5);
TreeNode *node6 = createNode(6);
TreeNode *node7 = createNode(7);
insertChild(root, node2);
insertChild(root, node3);
insertChild(node2, node4);
insertChild(node2, node5);
insertChild(node3, node6);
insertChild(node5, node7);
// 计算树的深度并输出结果
int depth = calculateDepth(root);
printf("树的深度为:%d\n", depth);
return 0;
}
```
在这个示例代码中,我们定义了一个`TreeNode`结构体表示树节点,并实现了`createNode`函数用于创建新节点,`insertChild`函数用于向节点添加子节点,以及`calculateDepth`函数用于递归计算树的深度。在`main`函数中,我们创建了一个孩子兄弟链表的树,并调用`calculateDepth`函数计算其深度,最后输出结果。
阅读全文
相关推荐

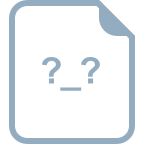
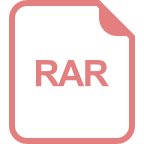
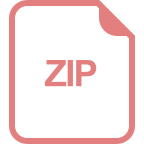
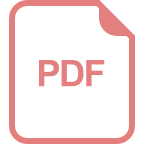
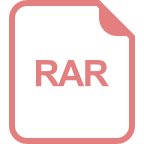
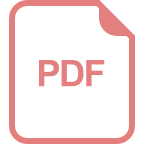
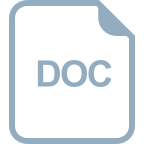
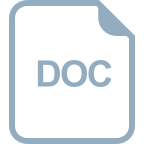
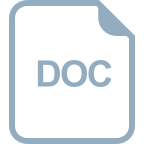
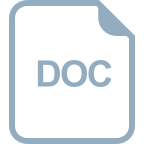
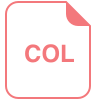

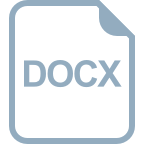