如何获取tkinter combobox选中的值在列表中的索引
时间: 2024-05-07 17:18:53 浏览: 10
可以使用`current()`方法获取combobox当前选中的值,再通过`index()`方法来获取该值在列表中的索引。具体代码如下:
```
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
# 创建一个列表和combobox
options = ["apple", "banana", "orange", "pear"]
combo = ttk.Combobox(root, values=options)
combo.pack()
def get_index():
# 获取选中的值的索引
selected_value = combo.current()
selected_index = options.index(selected_value)
print(selected_index)
button = tk.Button(root, text="Get Index", command=get_index)
button.pack()
root.mainloop()
```
当点击`Get Index`按钮时,会输出当前选中的值在列表中的索引。注意,`current()`方法返回的是选中值在combobox中的索引,而不是在列表中的索引,因此需要再用`options.index()`方法将选中值转换为在列表中的索引。
相关问题
tkinter combobox获取值
要获取tkinter combobox的值,可以使用get()方法。具体步骤如下:
1. 创建一个Combobox对象,将其绑定到窗口上。
2. 设置下拉框的内容。
3. 在需要获取值的地方,使用get()方法获取选中的值。
例如,如果我们有一个名为Comb的Combobox对象,我们可以使用以下代码获取选中的值:
selected_value = Comb.get()
python tkinter combobox如何获取选中的值
可以使用 `combobox.get()` 方法来获取当前选中的值。
以下是一个简单的示例代码:
```python
import tkinter as tk
from tkinter import ttk
def on_select(event):
selected_value = combobox.get()
print(selected_value)
root = tk.Tk()
options = ["Option 1", "Option 2", "Option 3"]
combobox = ttk.Combobox(root, values=options)
combobox.pack()
combobox.bind("<<ComboboxSelected>>", on_select)
root.mainloop()
```
在上面的示例中,我们使用 `combobox.bind` 方法将其与 `"<<ComboboxSelected>>"` 事件绑定起来。当用户选择新的选项时,`on_select` 函数将会被调用,并从 `combobox` 中获取当前选中的值并打印出来。
相关推荐
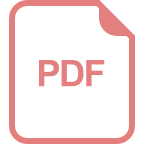












