给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出 和为目标值 target 的那 两个 整数,并返回它们的数组下标。 你可以假设每种输入只会对应一个答案。但是,数组中同一个元素在答案里不能重复出现。 你可以按任意顺序返回答案。 来源:力扣(LeetCode) 链接:https://leetcode.cn/problems/two-sum 著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
时间: 2024-04-10 15:29:24 浏览: 56
题目描述:给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出和为目标值 target 的那两个整数,并返回它们的数组下标。
解题思路:可以使用哈希表,对于每个元素,首先判断 target - x 是否在哈希表中,如果在,直接返回两个数的下标即可。如果不在,将这个数加入哈希表中。
Python 代码实现:
```
class Solution:
def twoSum(self, nums: List[int], target: int) -> List[int]:
hash_table = {}
for i, x in enumerate(nums):
if target - x in hash_table:
return [hash_table[target - x], i]
hash_table[x] = i
```
时间复杂度:O(n)。
空间复杂度:O(n)。
相关问题
给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出 和为目标值 target 的那 两个 整数,并返回它们的数组下标。
题目描述:
给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出和为目标值 target 的那两个整数,并返回它们的数组下标。
解题思路:
使用哈希表来存储每个元素的值和它的索引,然后遍历数组中的每个元素 x,查找是否存在一个值与 target - x 相等的目标元素。
算法流程:
1. 初始化一个空的哈希表。
2. 遍历数组中的每个元素 x。
3. 在哈希表中查找是否存在一个值与 target - x 相等的目标元素。
4. 如果存在,直接返回两个元素的下标。
5. 如果不存在,将当前元素的值和它的索引存储到哈希表中。
时间复杂度:O(n)
空间复杂度:O(n)
Python 代码实现:
class Solution:
def twoSum(self, nums: List[int], target: int) -> List[int]:
hashmap = {}
for i, num in enumerate(nums):
if target - num in hashmap:
return [hashmap[target - num], i]
hashmap[num] = i
return []
jsp给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出和为目标值 target 的那 两个 整数,并返回它们的数组下标
好的,根据您的要求,我理解你需要一个算法实现,以下是实现代码:
```java
public int[] twoSum(int[] nums, int target) {
Map<Integer, Integer> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
if (map.containsKey(complement)) {
return new int[] { map.get(complement), i };
}
map.put(nums[i], i);
}
throw new IllegalArgumentException("No two sum solution");
}
```
阅读全文
相关推荐
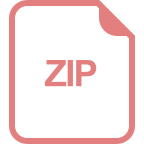
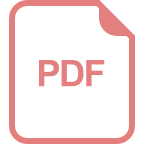
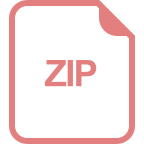



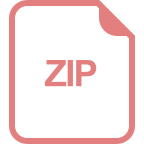








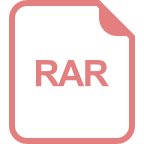