实现最佳适应算法代码构思
时间: 2023-09-18 09:13:55 浏览: 44
下面是一份 C++ 代码,实现了最佳适应算法:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct MemoryBlock {
int start_address; // 起始地址
int end_address; // 结束地址
int size; // 块大小
bool is_allocated; // 是否已分配
};
// 按照块大小从小到大排序
bool compare_blocks(MemoryBlock a, MemoryBlock b) {
return a.size < b.size;
}
// 最佳适应算法
void best_fit(vector<MemoryBlock>& memory, int process_size) {
// 按照块大小从小到大排序
sort(memory.begin(), memory.end(), compare_blocks);
// 查找最小但能够满足进程需求的块
for (int i = 0; i < memory.size(); i++) {
if (!memory[i].is_allocated && memory[i].size >= process_size) {
memory[i].is_allocated = true;
cout << "Process allocated to block starting at address " << memory[i].start_address << endl;
break;
}
}
}
int main() {
// 初始化内存块
vector<MemoryBlock> memory = {
{0, 99, 100, false},
{100, 199, 100, false},
{200, 299, 100, false},
{300, 399, 100, false},
{400, 499, 100, false}
};
// 分配进程
best_fit(memory, 50);
best_fit(memory, 150);
best_fit(memory, 200);
return 0;
}
```
这份代码首先定义了一个 `MemoryBlock` 结构体来表示内存块,然后定义了一个 `compare_blocks` 函数来按照块大小从小到大排序内存块。最后,`best_fit` 函数实现了最佳适应算法,遍历所有内存块并找到最小但能够满足进程需求的块进行分配。最后,在 `main` 函数中初始化了内存块,并分配了三个进程。
相关推荐
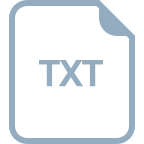














