c#取外部listview内容
时间: 2023-10-28 14:32:58 浏览: 78
你可以使用Windows API函数来获取外部ListView控件的内容。具体步骤如下:
1. 获取外部程序的窗口句柄,可以使用FindWindow或FindWindowEx等函数;
2. 获取ListView控件的句柄,可以使用FindWindowEx、GetDlgItem、GetTopWindow等函数;
3. 使用ListView_GetItemCount函数获取ListView控件中项的数量;
4. 使用ListView_GetItemText函数获取ListView控件中每一项的文本内容。
以下是一个示例代码:
```csharp
[DllImport("user32.dll")]
public static extern IntPtr FindWindow(string lpClassName, string lpWindowName);
[DllImport("user32.dll")]
public static extern IntPtr FindWindowEx(IntPtr hwndParent, IntPtr hwndChildAfter, string lpszClass, string lpszWindow);
[DllImport("user32.dll", CharSet = CharSet.Auto)]
public static extern int SendMessage(IntPtr hWnd, int Msg, int wParam, StringBuilder lParam);
[DllImport("user32.dll", CharSet = CharSet.Auto)]
public static extern IntPtr SendMessage(IntPtr hWnd, int Msg, int wParam, IntPtr lParam);
[DllImport("user32.dll", CharSet = CharSet.Auto)]
public static extern IntPtr GetDlgItem(IntPtr hDlg, int nIDDlgItem);
[DllImport("user32.dll", CharSet = CharSet.Auto)]
public static extern IntPtr GetTopWindow(IntPtr hWnd);
[DllImport("user32.dll", CharSet = CharSet.Auto)]
public static extern int ListView_GetItemCount(IntPtr hwnd);
[DllImport("user32.dll", CharSet = CharSet.Auto)]
public static extern int ListView_GetItemText(IntPtr hwnd, int i, int iSubItem, StringBuilder pszText, int cchTextMax);
public static string GetListViewItemText(IntPtr hwndListView, int index, int subIndex)
{
int pid;
GetWindowThreadProcessId(hwndListView, out pid);
IntPtr hProcess = OpenProcess(ProcessAccessFlags.All, false, pid);
int itemCount = ListView_GetItemCount(hwndListView);
if (index >= itemCount)
return "";
IntPtr result = IntPtr.Zero;
IntPtr pszText = IntPtr.Zero;
try
{
pszText = Marshal.AllocHGlobal(256 * 2);
SendMessage(hwndListView, 0x1000 + 75, index, 0); // LVM_ENSUREVISIBLE
SendMessage(hwndListView, 0x1000 + 19, index, 0x0001); // LVM_SELECTITEM
SendMessage(hwndListView, 0x1000 + 15, index, 0); // LVM_GETITEMRECT
SendMessage(hwndListView, 0x1000 + 58, 0, MakeLParam(0, 0x0001)); // LVM_GETSUBITEMRECT
SendMessage(hwndListView, 0x1000 + 187, index * 0x10000 + subIndex, 0); // LVM_GETITEMTEXT
SendMessage(hwndListView, 0x1000 + 14, 0, MakeLParam(0, 0)); // LVM_SCROLL
int cch = SendMessage(hwndListView, 0x1000 + 76, index, pszText);
if (cch > 0)
{
StringBuilder text = new StringBuilder(cch + 1);
SendMessage(hwndListView, 0x1000 + 45, index, text);
return text.ToString();
}
}
finally
{
if (pszText != IntPtr.Zero)
Marshal.FreeHGlobal(pszText);
CloseHandle(hProcess);
}
return "";
}
public static IntPtr MakeLParam(int loWord, int hiWord)
{
return (IntPtr)(((ushort)loWord) | (hiWord << 16));
}
// Usage
IntPtr hwnd = FindWindow("Notepad", null);
IntPtr hwndEdit = FindWindowEx(hwnd, IntPtr.Zero, "Edit", null);
IntPtr hwndList = GetDlgItem(hwnd, 0x3E9);
string text = GetListViewItemText(hwndList, 0, 1);
```
这里的示例代码是用于获取Notepad中的ListView控件的第一行第二列的文本内容。你需要根据具体情况修改代码中的窗口类名、控件ID等参数。
阅读全文
相关推荐
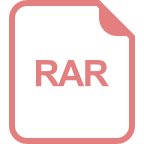



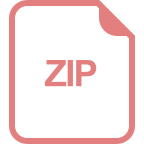
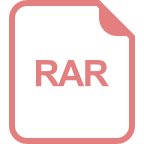









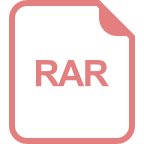
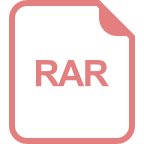