利用Java实现词频统计
时间: 2024-05-16 18:17:46 浏览: 25
实现词频统计的一种简单方法是使用Java中的HashMap数据结构,将每个单词作为键,出现次数作为值。具体实现步骤如下:
1. 读取文本文件,将文件内容存储在一个字符串中。
2. 使用正则表达式将字符串中的非字母字符替换为空格,以便将文本分割成单词。
```java
String content = readFileAsString("input.txt"); // 读取文本文件
content = content.replaceAll("[^a-zA-Z]", " "); // 将非字母字符替换为空格
```
3. 将文本分割成单词,并统计每个单词出现的次数。
```java
Map<String, Integer> wordCount = new HashMap<>();
String[] words = content.split("\\s+");
for (String word : words) {
word = word.toLowerCase(); // 将单词转换为小写字母,以便不区分大小写
if (!word.isEmpty()) {
int count = wordCount.getOrDefault(word, 0);
wordCount.put(word, count + 1);
}
}
```
4. 输出每个单词及其出现次数。
```java
for (String word : wordCount.keySet()) {
int count = wordCount.get(word);
System.out.println(word + ": " + count);
}
```
完整代码如下:
```java
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.HashMap;
import java.util.Map;
public class WordCount {
public static void main(String[] args) throws IOException {
String content = readFileAsString("input.txt"); // 读取文本文件
content = content.replaceAll("[^a-zA-Z]", " "); // 将非字母字符替换为空格
Map<String, Integer> wordCount = new HashMap<>();
String[] words = content.split("\\s+");
for (String word : words) {
word = word.toLowerCase(); // 将单词转换为小写字母,以便不区分大小写
if (!word.isEmpty()) {
int count = wordCount.getOrDefault(word, 0);
wordCount.put(word, count + 1);
}
}
for (String word : wordCount.keySet()) {
int count = wordCount.get(word);
System.out.println(word + ": " + count);
}
}
private static String readFileAsString(String filePath) throws IOException {
return new String(Files.readAllBytes(Paths.get(filePath)));
}
}
```
其中,`readFileAsString`方法用于读取文本文件并返回字符串。如果需要统计多个文件的词频,可以将整个统计过程放到一个循环中,每次读取一个文件并更新词频统计结果。
相关推荐
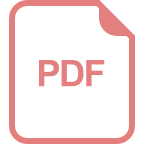














