fcos 后处理 c++代码实现
时间: 2023-12-16 07:03:44 浏览: 151
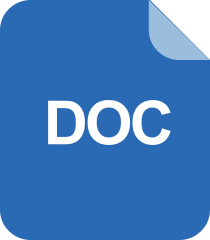
c++代码的实现
FCOS(Fully Convolutional One-Stage Object Detection)是一种目标检测算法,其后处理部分的C++代码实现可以参考以下步骤:
1. 对检测结果进行筛选和排序。可以根据置信度对检测结果进行排序,并设置置信度阈值,只保留置信度高于阈值的检测结果。
2. 进行非极大值抑制(NMS)。NMS可以去除重复的检测结果,将重复区域的检测结果合并为一个,只保留置信度最高的检测结果。
3. 进行边界框回归。将检测结果中的边界框根据预测的偏移量进行调整,得到更精确的边界框位置。
下面是一个简单的FCOS后处理C++代码实现示例:
```c++
void fcos_post_process(float* scores, float* bbox_regs, float* centerness, int num_anchors, int num_classes, int img_width, int img_height, float score_thresh, float nms_thresh, vector<DetectedObject>& detected_objects)
{
vector<DetectedObject> all_objects;
for (int i = 0; i < num_anchors; i++)
{
for (int j = 0; j < num_classes; j++)
{
int idx = i * num_classes + j;
float score = sigmoid(scores[idx]);
if (score > score_thresh)
{
float cx = (float)i / num_anchors * img_width;
float cy = (float)j / num_classes * img_height;
float w = bbox_regs[idx * 4];
float h = bbox_regs[idx * 4 + 1];
float x1 = cx - w / 2;
float y1 = cy - h / 2;
float x2 = cx + w / 2;
float y2 = cy + h / 2;
float centerness_score = sigmoid(centerness[idx]);
DetectedObject obj;
obj.bbox = BBox(x1, y1, x2, y2);
obj.score = score * centerness_score;
obj.class_id = j;
all_objects.push_back(obj);
}
}
}
sort(all_objects.begin(), all_objects.end(), [](const DetectedObject& a, const DetectedObject& b) {
return a.score > b.score;
});
vector<DetectedObject> nms_objects;
for (auto& obj : all_objects)
{
bool keep = true;
for (auto& nms_obj : nms_objects)
{
if (iou(obj.bbox, nms_obj.bbox) > nms_thresh)
{
keep = false;
break;
}
}
if (keep)
{
nms_objects.push_back(obj);
}
}
detected_objects = nms_objects;
}
```
这段代码实现了FCOS算法的后处理部分,其中sigmoid函数用于将输出值转换为概率值,iou函数用于计算两个边界框之间的IoU(Intersection over Union)。最终得到的检测结果保存在detected_objects数组中。
阅读全文
相关推荐
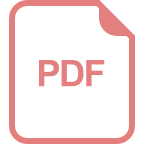
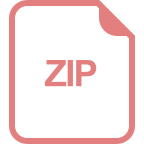

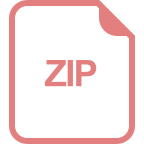
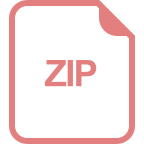
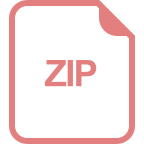
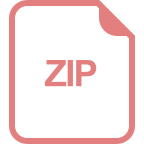
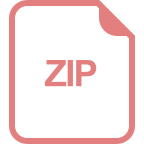
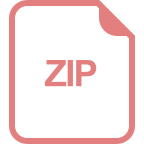
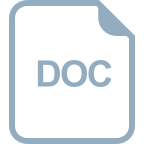
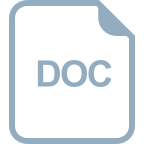
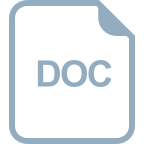


