java spring boot memcached 增删改查
时间: 2024-06-22 16:01:40 浏览: 213
在Java Spring Boot中使用Memcached作为缓存技术,可以帮助提高应用性能,通过减少数据库访问次数。以下是Spring Boot集成Memcached的基本操作:
1. 添加依赖:首先,你需要在你的`pom.xml`文件中添加Spring Data Memcached和Memcached客户端库的依赖。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>net.spy</groupId>
<artifactId>spymemcached</artifactId>
</dependency>
```
2. 配置 Memcached:在`application.properties`或`application.yml`中添加Memcached的配置,例如主机名和端口。
```properties
spring.data.memcached.servers=localhost:11211
```
3. 使用`@Cacheable`注解进行缓存读取(增):
在方法上添加`@Cacheable`,Spring会尝试从Memcached获取数据,如果存在则返回,不存在则执行实际的方法并存入缓存。
```java
@GetMapping("/cache")
@Cacheable("myCacheKey")
public String fetchData() {
// 实际的业务逻辑
}
```
4. 使用`@CachePut`注解进行缓存更新(改):
如果你想更新缓存中的值,可以使用`@CachePut`。这会在缓存中找到指定键的值后,执行你的更新操作。
```java
@PostMapping("/update")
@CachePut(value = "myCacheKey", key = "#id")
public void updateData(@RequestBody MyEntity entity) {
// 更新数据逻辑
}
```
5. 使用`@CacheEvict`注解进行缓存删除(删):
如果需要从缓存中移除某个键的数据,可以使用`@CacheEvict`。
```java
@DeleteMapping("/delete")
@CacheEvict(value = "myCacheKey", key = "#id")
public void deleteData(@PathVariable Long id) {
// 删除数据逻辑
}
```
6. 使用`@CacheConfig`和`@Caching`进行批量操作(查):
如果需要对多个键进行缓存操作,可以使用`@Caching`注解,并在`@CacheConfig`中定义缓存策略。
```java
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
return new SimpleCacheManager(Arrays.asList(
new RedisCacheConfiguration("myOtherCache")));
}
}
@GetMapping("/batchFetch")
@Caching(caches = {"myOtherCache"})
public List<String> fetchManyData() {
// 执行多个查询操作
}
```
阅读全文
相关推荐
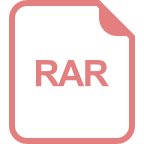
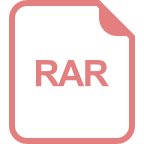
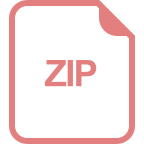















