对pycharm程序进行显存限制和算力限制
时间: 2024-02-26 21:57:56 浏览: 22
对于 PyCharm,它是一个集成开发环境 (IDE),并不直接涉及显存和算力的限制。但是,如果你想限制某个 Python 程序的显存和算力,可以通过以下方法实现:
1. 对于显存限制,你可以使用 TensorFlow、PyTorch 等框架中的 `tf.config.gpu.set_per_process_memory_fraction` 或 `torch.cuda.set_per_process_memory_fraction` 方法,将显存使用限制在一定的比例内。例如,以下代码将限制 TensorFlow 使用的显存为 GPU 总显存的 60%:
```python
import tensorflow as tf
gpus = tf.config.list_physical_devices('GPU')
if gpus:
# Restrict TensorFlow to only allocate 60% of the GPU memory
try:
for gpu in gpus:
tf.config.experimental.set_memory_growth(gpu, True)
tf.config.experimental.set_virtual_device_configuration(
gpu, [tf.config.experimental.VirtualDeviceConfiguration(memory_limit=0.6)])
logical_gpus = tf.config.experimental.list_logical_devices('GPU')
print(len(gpus), "Physical GPUs,", len(logical_gpus), "Logical GPUs")
except RuntimeError as e:
# Memory growth must be set before GPUs have been initialized
print(e)
```
2. 对于算力限制,你可以使用 `multiprocessing` 模块中的 `Process` 类和 `setrlimit` 方法,将 CPU 使用限制在一定的时间和空间内。例如,以下代码将限制某个函数的运行时间为 10 秒:
```python
import multiprocessing
import resource
def my_function():
# Your function here
pass
if __name__ == '__main__':
# Set time limit to 10 seconds
resource.setrlimit(resource.RLIMIT_CPU, (10, 10))
p = multiprocessing.Process(target=my_function)
p.start()
p.join()
```
请注意,以上方法均为 Python 代码级别的限制,仍然无法完全保证显存和算力的安全。如果需要更严格的限制,可以考虑使用 Docker、Kubernetes 等容器技术,或者直接在操作系统级别进行限制。
相关推荐
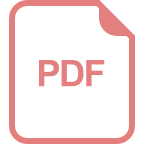
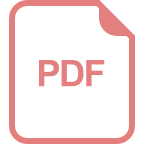














