使用sigar监听指定目录文件变化
时间: 2024-06-10 07:09:53 浏览: 12
Sigar是Java的一个跨平台系统信息库,可以获取系统的各种信息,包括CPU、内存、磁盘、网络等等。但是,它并没有提供监听文件变化的功能,可以使用Java的WatchService实现文件变化的监听。
1. 首先,需要引入Sigar的依赖包。可以从官网(https://www.hyperic.com/products/sigar)下载最新版本的Sigar,解压后将其中的jar包引入项目中。
2. 创建一个监控类,实现WatchService的功能,用于监听指定目录下的文件变化。
```java
import java.io.IOException;
import java.nio.file.*;
import java.util.List;
public class FileMonitor {
private static WatchService watchService;
private static Path path;
public static void main(String[] args) throws IOException, InterruptedException {
String dir = "D:/test";
path = Paths.get(dir);
watchService = path.getFileSystem().newWatchService();
// 监听ENTRY_CREATE、ENTRY_MODIFY、ENTRY_DELETE事件
path.register(watchService, StandardWatchEventKinds.ENTRY_CREATE, StandardWatchEventKinds.ENTRY_MODIFY, StandardWatchEventKinds.ENTRY_DELETE);
System.out.println("监控目录:" + dir);
while (true) {
WatchKey watchKey = watchService.take();
List<WatchEvent<?>> watchEvents = watchKey.pollEvents();
for (WatchEvent<?> watchEvent : watchEvents) {
WatchEvent.Kind<?> kind = watchEvent.kind();
if (kind == StandardWatchEventKinds.OVERFLOW) {
continue;
}
// 文件路径
Path watchPath = (Path) watchEvent.context();
String filePath = dir + "\\" + watchPath.toString();
// 处理文件变化
System.out.println("文件变化:" + filePath);
}
// 重置监控器
boolean valid = watchKey.reset();
if (!valid) {
break;
}
}
}
}
```
3. 在处理文件变化的代码中,可以使用Sigar获取文件的信息。
```java
// 处理文件变化
System.out.println("文件变化:" + filePath);
// 获取文件信息
Sigar sigar = new Sigar();
FileSystem fs = FileSystemUtils.getFileSystem(sigar, path.toString());
FileInfo fileInfo = fs.getFileInfo(filePath);
System.out.println("文件大小:" + fileInfo.getSize());
System.out.println("文件修改时间:" + new Date(fileInfo.getMTime()));
```
4. 运行程序,在指定目录下新建、修改、删除文件,可以看到控制台输出相应的文件变化信息和文件信息。
```console
监控目录:D:/test
文件变化:D:/test\test.txt
文件大小:12
文件修改时间:Fri May 28 11:36:30 CST 2021
文件变化:D:/test\test.txt
文件大小:15
文件修改时间:Fri May 28 11:36:46 CST 2021
文件变化:D:/test\test.txt
文件大小:15
文件修改时间:Fri May 28 11:37:02 CST 2021
文件变化:D:/test\test.txt
文件大小:12
文件修改时间:Fri May 28 11:37:13 CST 2021
```
相关推荐
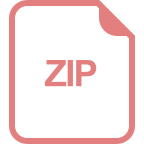














