编写C sharp复制文件的代码
时间: 2023-09-23 08:05:20 浏览: 148
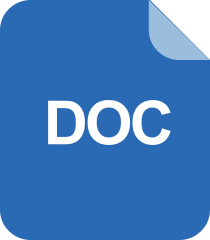
复制文件C代码

### 回答1:
可以使用以下代码来复制文件:
```csharp
using System.IO;
class Program
{
static void Main(string[] args)
{
string sourceFile = @"C:\source\file.txt";
string destinationFile = @"C:\destination\file.txt";
// To copy a file to another location and
// overwrite the destination file if it already exists.
File.Copy(sourceFile, destinationFile, true);
}
}
```
其中,`sourceFile` 是源文件的路径,`destinationFile` 是目标文件的路径。`File.Copy` 方法会将源文件复制到目标文件,并且如果目标文件已经存在,则会覆盖它。
### 回答2:
下面是一个用C#编写的复制文件的代码示例:
```
using System;
using System.IO;
class Program
{
static void Main(string[] args)
{
string sourceFilePath = "source.txt"; // 源文件路径
string destinationFilePath = "destination.txt"; // 目标文件路径
try
{
// 创建一个新的文件流读取源文件
using (FileStream sourceStream = new FileStream(sourceFilePath, FileMode.Open))
{
// 创建一个新的文件流写入目标文件
using (FileStream destinationStream = new FileStream(destinationFilePath, FileMode.Create))
{
// 将源文件内容复制到目标文件中
sourceStream.CopyTo(destinationStream);
Console.WriteLine("文件复制完成");
}
}
}
catch (IOException e)
{
Console.WriteLine($"文件复制出错: {e.Message}");
}
Console.ReadLine();
}
}
```
以上代码中,我们首先指定了源文件路径和目标文件路径。然后尝试打开源文件,创建一个新的文件流进行读取。接着创建一个用于写入的新的文件流。然后使用`CopyTo`方法,将源文件的内容复制到目标文件中。最后,我们在控制台输出一个表示文件复制完成的信息。如果复制过程中出现任何错误,我们将捕获并显示异常消息。
请注意,在运行此代码之前,请确保源文件存在且可读,并且目标文件的目录存在且可写。
### 回答3:
下面是一个使用C#编写的复制文件的代码示例:
```csharp
using System;
using System.IO;
class Program
{
static void Main(string[] args)
{
string sourceFilePath = "C:\\source\\file.txt";
string destinationFilePath = "C:\\destination\\file.txt";
try
{
// 检查源文件是否存在
if (!File.Exists(sourceFilePath))
{
throw new FileNotFoundException("源文件不存在");
}
// 复制文件
File.Copy(sourceFilePath, destinationFilePath, true);
Console.WriteLine("文件复制成功");
}
catch (IOException ex)
{
Console.WriteLine("文件复制时发生IO错误:" + ex.Message);
}
catch (Exception ex)
{
Console.WriteLine("文件复制时发生错误:" + ex.Message);
}
Console.ReadLine();
}
}
```
这段代码首先指定了源文件和目标文件的路径。然后,通过检查源文件是否存在,确保复制操作可以进行。接着,调用`File.Copy`方法进行文件复制。第三个参数`true`表示如果目标文件已经存在,则覆盖它。最后,在`try-catch`块中处理可能出现的异常,并给出相应的错误提示。
注意,此示例仅演示了基本的文件复制操作,如需处理更复杂的情况(例如复制文件夹),可能需要使用其他方法或库。
阅读全文
相关推荐
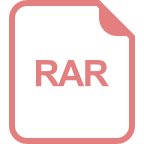
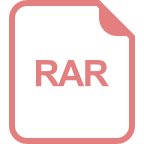
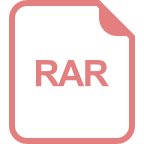
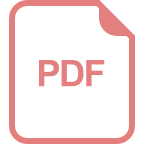
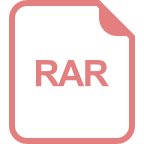
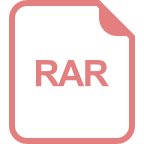
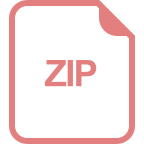
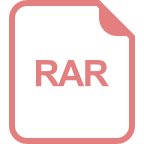
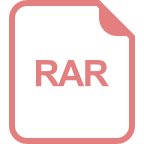
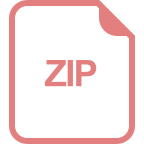
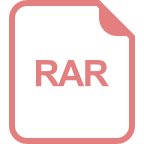
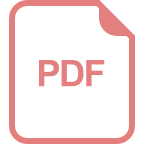
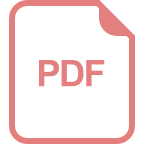
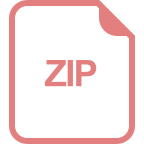
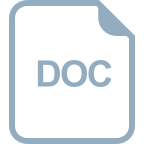
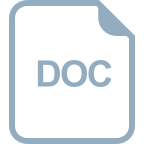