请写一个C++程序,递归遍历文件夹“./resources”下的所有.txt后缀的文本文件,并将含有字符串"Hello World"的文本文件内容以utf-8编码格式,一行一个文件地输出到控制台上
时间: 2024-03-21 21:43:33 浏览: 56
以下是一个递归遍历文件夹并搜索文件内容的示例程序:
```c++
#include <iostream>
#include <fstream>
#include <filesystem>
void search_and_print(const std::filesystem::path& path) {
if (std::filesystem::is_directory(path)) { // 如果是文件夹,则递归遍历子文件夹
for (const auto& sub_path : std::filesystem::directory_iterator(path)) {
search_and_print(sub_path);
}
} else if (path.extension() == ".txt") { // 如果是txt文件,则搜索文件内容
std::ifstream ifs(path, std::ios::in | std::ios::binary);
if (ifs.is_open()) {
ifs.seekg(0, std::ios::end);
std::streamoff size = ifs.tellg();
ifs.seekg(0, std::ios::beg);
std::string content(size, '\0');
ifs.read(&content[0], size);
ifs.close();
if (content.find("Hello World") != std::string::npos) { // 如果文件内容包含"Hello World",则输出到控制台
std::cout << "File: " << path << std::endl;
std::cout << content << std::endl;
}
}
}
}
int main() {
std::filesystem::path folder_path("./resources");
search_and_print(folder_path);
return 0;
}
```
注意,以上代码仅在C++17及以上的标准下可用,因为它使用了C++17新增的文件系统库。
阅读全文
相关推荐
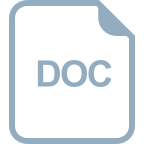
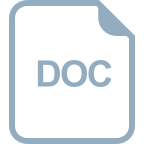
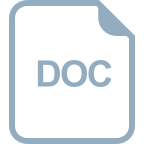
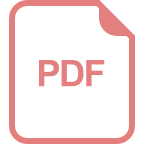
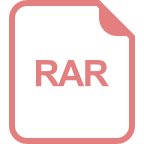
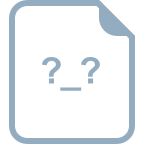
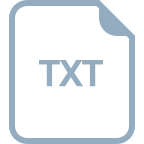
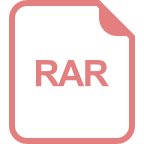
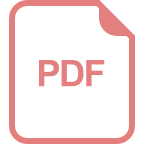
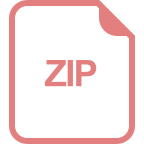
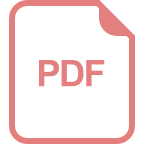
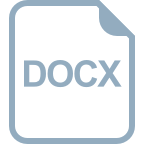
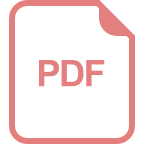
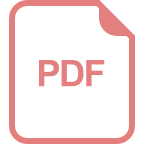