六轴工业机器人正逆解算法c代码
时间: 2023-05-13 15:03:29 浏览: 929
六轴工业机器人正逆解算法的c代码主要包括两个部分:正解算法和逆解算法。
正解算法主要用于计算机器人每个关节的运动轨迹,将机器人末端执行器沿给定的路径运动。其核心在于通过正弦余弦函数求解机器人每个关节的角度,从而得到机器人的运动轨迹。其c代码如下:
void forward_kinematics(double *theta, double *T)
{
// 正向运动学计算机器人末端位形(位置和姿态)
double link[6] = {0.0, 0.0, 0.0, 0.0, 0.0, 0.0};
double R[3][3];
double Px, Py, Pz;
double a[6] = {0, L2, L3, 0, 0, 0};
double d[6] = {L1, 0, 0, L4, L5, L6};
double alpha[6] = {-PI/2, 0, 0, PI/2, -PI/2, 0};
double temp_theta[6];
for(int i = 0; i < 6; i++) {
temp_theta[i] = theta[i];
}
double T06[4][4] = {0};
for (int i = 0; i < 6; i++) {
double theta = temp_theta[i] + offset[i];
double s_theta = sin(theta);
double c_theta = cos(theta);
double s_alpha = sin(alpha[i]);
double c_alpha = cos(alpha[i]);
link[i] = c_theta;
if (i == 1) {
link[i] = s_theta;
}
if (i < 3) {
Px += a[i] * c_theta;
Py += a[i] * s_theta;
Pz += d[i];
} else {
R[0][0] = (i == 3) ? c_theta : -s_theta;
R[0][1] = -c_alpha * s_theta;
R[0][2] = s_alpha * s_theta;
R[1][0] = (i == 3) ? s_theta : c_theta;
R[1][1] = c_alpha * c_theta;
R[1][2] = -s_alpha * c_theta;
R[2][0] = 0;
R[2][1] = s_alpha;
R[2][2] = c_alpha;
double Px_ = a[i] * (R[0][0] * link[3] + R[0][1] * link[4] + R[0][2] * link[5]);
double Py_ = a[i] * (R[1][0] * link[3] + R[1][1] * link[4] + R[1][2] * link[5]);
double Pz_ = a[i] * (R[2][0] * link[3] + R[2][1] * link[4] + R[2][2] * link[5]);
Px += Px_;
Py += Py_;
Pz += Pz_;
}
}
T06[0][0] = R[0][0]; T06[0][1] = R[0][1]; T06[0][2] = R[0][2]; T06[0][3] = Px;
T06[1][0] = R[1][0]; T06[1][1] = R[1][1]; T06[1][2] = R[1][2]; T06[1][3] = Py;
T06[2][0] = R[2][0]; T06[2][1] = R[2][1]; T06[2][2] = R[2][2]; T06[2][3] = Pz;
T06[3][0] = 0; T06[3][1] = 0; T06[3][2] = 0; T06[3][3] = 1;
memcpy(T, T06, sizeof(T06));
}
逆解算法主要用于将机器人末端执行器的位姿转换为对应的关节角度,从而实现机器人的运动姿态。其核心在于通过使用dh参数确定机器人各关节的初始角度,然后用逆算法求解机器人末端执行器的位姿。其c代码如下:
int inverse_kinematics(double *T, double *theta_out)
{
double theta1,theta2,theta3,theta4,theta5,theta6;
double Px,Py,Pz;
double a[6] = {0, L2, L3, 0, 0, 0};
double d[6] = {L1, 0, 0, L4, L5, L6};
double alpha[6] = {-PI/2, 0, 0, PI/2, -PI/2, 0};
Px = T[0*4+3];
Py = T[1*4+3];
Pz = T[2*4+3];
double phi = atan2(Py,Px);
double L7 = sqrt(Px*Px+Py*Py) - a[1];
double L8 = Pz - d[0];
double L9 = sqrt(L7*L7+L8*L8);
if (L9 < (L3+L4+L5+L6)) {
theta1 = phi + PI / 2;
theta3 = acos((L9*L9-L3*L3-L4*L4-L5*L5-L6*L6)/(2*L3*L4));
double a1,a2,a3,a4,a5,a6,b1,b2,b3,b4,b5,b6,c1,c2,c3,c4,c5,c6;
a1 = L4*cos(theta3)+L3;
b1 = L4*sin(theta3);
c1 = L8;
double a2_,b2_,c2_;
a2_ = a1*cos(alpha[1])+c1*sin(alpha[1]);
b2_ = b1;
c2_ = -a1*sin(alpha[1])+c1*cos(alpha[1]);
a2 = a2_;
b2 = b2_+a[2];
c2 = c2_+d[1];
double L10 = sqrt(a2*a2+b2*b2+c2*c2);
if ((theta3 > 0) && (theta3 < PI)) {
theta2 = atan2(b2,c2) - atan2((a2*a2+b2*b2+c2*c2-L2*L2)/(2*L2),sqrt(1-((a2*a2+b2*b2+c2*c2-L2*L2)/(2*L2))*((a2*a2+b2*b2+c2*c2-L2*L2)/(2*L2))));
} else {
theta2 = atan2(b2,c2) + atan2(sqrt(1-((a2*a2+b2*b2+c2*c2-L2*L2)/(2*L2))*((a2*a2+b2*b2+c2*c2-L2*L2)/(2*L2))),(a2*a2+b2*b2+c2*c2-L2*L2)/(2*L2));
}
double a3_,b3_,c3_;
a3_ = a2*cos(theta2)+c2*sin(theta2);
b3_ = b2;
c3_ = -a2*sin(theta2)+c2*cos(theta2);
a3 = a3_*cos(alpha[2])+c3_*sin(alpha[2]);
b3 = b3_;
c3 = -a3_*sin(alpha[2])+c3_*cos(alpha[2]);
double a4_,b4_,c4_;
a4_ = a3*cos(theta3-theta2)+c3*sin(theta3-theta2);
b4_ = b3;
c4_ = -a3*sin(theta3-theta2)+c3*cos(theta3-theta2);
a4 = a4_*cos(alpha[3])+c4_*sin(alpha[3]);
b4 = b4_;
c4 = -a4_*sin(alpha[3])+c4_*cos(alpha[3]);
theta4 = atan2(b4,c4);
double a5_,b5_,c5_;
a5_ = a4*cos(alpha[4])+b4*sin(alpha[4]);
b5_ = -a4*sin(alpha[4])+b4*cos(alpha[4]);
c5_ = c4;
double a5 = a5_;
double b5 = b5_;
double L11 = sqrt(a5*a5+b5*b5+c5*c5);
double L12 = sqrt(a5*a5+b5*b5);
if (theta4 > 0) {
theta5 = atan2(-L12,c5);
} else {
theta5 = atan2(L12,c5);
}
double a6_,b6_,c6_;
a6_ = a5*cos(alpha[5])+b5*sin(alpha[5]);
b6 = -a5*sin(alpha[5])+b5*cos(alpha[5]);
c6_ = c5;
a6 = a6_*cos(theta5)+b6*sin(theta5);
c6 = -a6_*sin(theta5)+c6_*cos(theta5);
theta6 = atan2(-b6,c6);
theta_out[0] = theta1 - offset[0];
theta_out[1] = theta2 - offset[1];
theta_out[2] = theta3 - offset[2];
theta_out[3] = theta4 - offset[3];
theta_out[4] = theta5 - offset[4];
theta_out[5] = theta6 - offset[5];
return 1;
} else {
return 0;
}
}
阅读全文
相关推荐
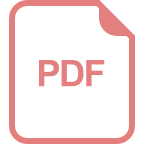
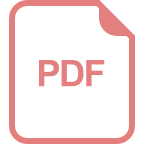
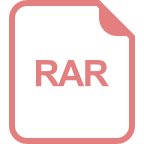

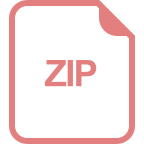
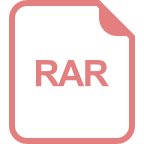
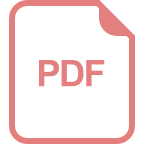
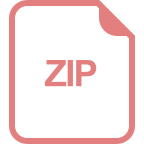
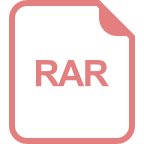
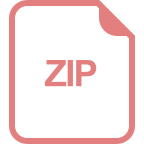
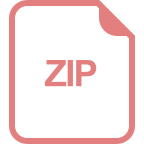
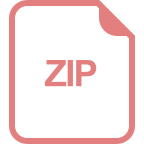
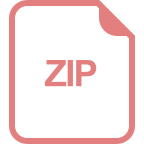
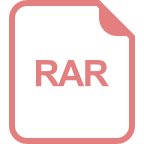
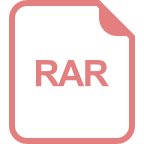
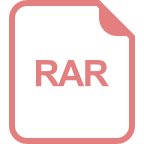
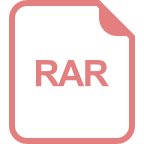
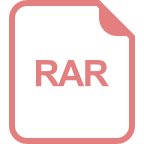