python我想对轮廓做极值点进行亚像素检测,获取更精确的左极值点
时间: 2024-02-13 16:04:30 浏览: 152
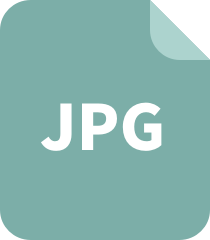
轮廓特征点检测

对于轮廓的极值点亚像素检测,可以使用 `cv2.findContours()`函数获取轮廓,然后对每个轮廓进行处理。具体实现步骤如下:
1. 读入二值化后的图像,使用 `cv2.findContours()` 函数获取轮廓。
2. 对于每个轮廓,使用 `cv2.approxPolyDP()` 函数对轮廓进行多边形拟合,得到拟合后的多边形顶点。
3. 对于多边形顶点,使用 `cv2.cornerSubPix()` 函数进行亚像素级别的角点检测,得到更加精确的顶点坐标。
4. 对于左极值点,通过比较左侧和右侧的像素值,确定其位置。
下面是一个简单的示例代码:
```python
import cv2
import numpy as np
# 读取二值化后的图像
img = cv2.imread('image.jpg', 0)
# 获取轮廓
_, contours, _ = cv2.findContours(img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 亚像素级别的角点检测
def refine_corners(corners):
criteria = (cv2.TERM_CRITERIA_EPS + cv2.TERM_CRITERIA_MAX_ITER, 30, 0.001)
sub_corners = cv2.cornerSubPix(img, corners, (5, 5), (-1, -1), criteria)
return sub_corners
# 对每个轮廓进行处理
for contour in contours:
# 多边形拟合
approx = cv2.approxPolyDP(contour, 3, True)
approx = np.squeeze(approx)
# 亚像素级别的角点检测
sub_corners = refine_corners(approx)
# 确定左极值点
leftmost = tuple(contour[contour[:,:,0].argmin()][0])
for i in range(len(sub_corners)):
if sub_corners[i][0] == leftmost[0]:
if img[sub_corners[i][1], sub_corners[i][0]-1] > img[sub_corners[i][1], sub_corners[i][0]+1]:
leftmost = (sub_corners[i][0]+1, sub_corners[i][1])
else:
leftmost = (sub_corners[i][0]-1, sub_corners[i][1])
# 显示轮廓和极值点
img_color = cv2.cvtColor(img, cv2.COLOR_GRAY2BGR)
cv2.drawContours(img_color, [approx], 0, (0, 255, 0), 2)
cv2.circle(img_color, leftmost, 3, (0, 0, 255), -1)
cv2.imshow('image', img_color)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码通过 `cv2.findContours()` 函数获取轮廓,然后对每个轮廓进行多边形拟合和亚像素级别的角点检测。对于左极值点,通过比较左侧和右侧的像素值确定其位置,最后在图像中显示轮廓和极值点。
阅读全文
相关推荐
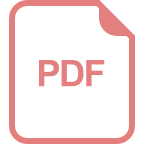
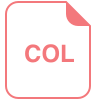
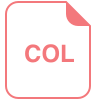
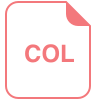
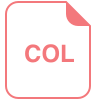
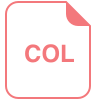
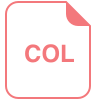
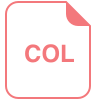
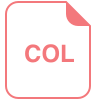

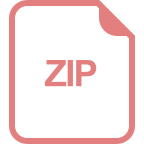
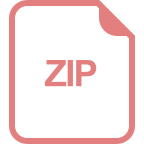
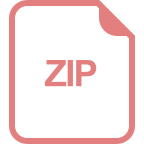
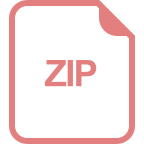
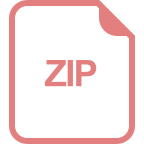