#ifndef MYAPP_H #define MYAPP_H #include <QObject> #include <QDebug> #include <QString> #include "mylogin.h" #include "myenroll.h" #include "tips.h" #include "chatinterface.h" #include "sqoperator.h" class myapp : public QObject { Q_OBJECT public: explicit myapp(QObject *parent = nullptr); SqOperator *mydb; //数据库类 mylogin * login; myenroll * enroll; tips * tip;//提示信息类 chatInterface *face;//主界面信息类 signals: public slots: bool judge(QString usrname, QString usrpass); void show_enroll_face(); void insertdb(QString usrname,QString usrpass); };解释每一段代码
时间: 2024-03-18 13:40:48 浏览: 62
这段代码是一个名为 `myapp` 的类的定义,继承自 `QObject` 类。它包含了一些头文件和一些成员变量和函数。以下是每一段代码的解释:
```c++
#ifndef MYAPP_H
#define MYAPP_H
#include <QObject>
#include <QDebug>
#include <QString>
#include "mylogin.h"
#include "myenroll.h"
#include "tips.h"
#include "chatinterface.h"
#include "sqoperator.h"
```
这部分代码是头文件的预处理指令,避免重复包含同一个头文件。
```c++
class myapp : public QObject {
Q_OBJECT
public:
explicit myapp(QObject *parent = nullptr);
SqOperator *mydb; //数据库类
mylogin * login;
myenroll * enroll;
tips * tip; //提示信息类
chatInterface *face; //主界面信息类
signals:
public slots:
bool judge(QString usrname, QString usrpass);
void show_enroll_face();
void insertdb(QString usrname,QString usrpass);
};
```
这部分定义了一个名为 `myapp` 的类,它继承自 `QObject` 类。类中包含了一些成员变量和函数:
1. `mydb`:类型为 `SqOperator*`,是一个数据库类指针。
2. `login`:类型为 `mylogin*`,是一个登录类指针。
3. `enroll`:类型为 `myenroll*`,是一个注册类指针。
4. `tip`:类型为 `tips*`,是一个提示信息类指针。
5. `face`:类型为 `chatInterface*`,是一个主界面信息类指针。
6. `judge(QString usrname, QString usrpass)`:返回值为 `bool` 类型的函数,用于验证用户名和密码是否正确。
7. `show_enroll_face()`:无返回值的函数,用于显示注册界面。
8. `insertdb(QString usrname,QString usrpass)`:无返回值的函数,用于将用户信息插入到数据库中。
```c++
#endif // MYAPP_H
```
这部分代码是头文件的结尾标记,避免重复包含同一个头文件。
阅读全文
相关推荐
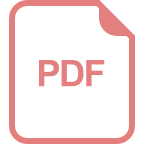
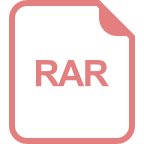















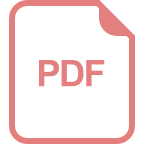