c++实现多线程的二进制文件和十六进制文件的相互转化工具
时间: 2023-11-27 17:50:26 浏览: 148
以下是一个简单的C++程序,可以实现二进制文件和十六进制文件的相互转换。该程序使用了多线程来提高转换速度。
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
#include <thread>
#include <mutex>
using namespace std;
mutex mtx; // 互斥锁,用于线程安全输出
void binaryToHex(const string& inputFileName, const string& outputFileName) {
ifstream inputFile(inputFileName, ios::binary);
ofstream outputFile(outputFileName);
stringstream ss;
ss << hex << uppercase << noshowbase << noskipws;
char c;
while (inputFile >> c) {
ss << static_cast<int>(c);
}
string hexString = ss.str();
for (int i = 0; i < hexString.length(); i += 2) {
string byteString = hexString.substr(i, 2);
outputFile << byteString << " ";
}
mtx.lock();
cout << "Binary to hex conversion completed!" << endl;
mtx.unlock();
}
void hexToBinary(const string& inputFileName, const string& outputFileName) {
ifstream inputFile(inputFileName);
ofstream outputFile(outputFileName, ios::binary);
stringstream ss;
string hexString;
while (inputFile >> hexString) {
int byte = stoi(hexString, nullptr, 16);
outputFile << static_cast<char>(byte);
}
mtx.lock();
cout << "Hex to binary conversion completed!" << endl;
mtx.unlock();
}
int main() {
string inputFileName = "input.bin";
string hexOutputFileName = "output.hex";
string binaryOutputFileName = "output.bin";
thread t1(binaryToHex, inputFileName, hexOutputFileName);
thread t2(hexToBinary, hexOutputFileName, binaryOutputFileName);
t1.join();
t2.join();
return 0;
}
```
该程序使用了两个函数 `binaryToHex` 和 `hexToBinary` 来实现二进制文件和十六进制文件的相互转换。这两个函数分别将输入文件转换成字符串,然后进行转换,并输出到对应的输出文件中。
在 `main` 函数中,我们创建了两个线程 `t1` 和 `t2` 分别用来执行转换函数。使用 `join` 方法等待线程执行完毕后,程序退出。
注意,在输出结果时,我们使用了一个互斥锁 `mtx` 来保证线程安全输出。
阅读全文
相关推荐
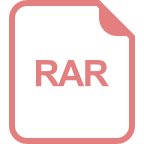
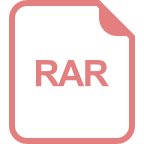


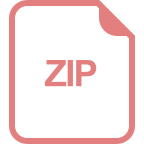
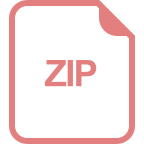
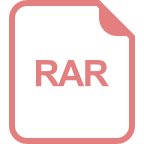
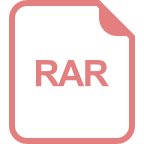
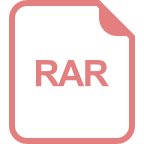
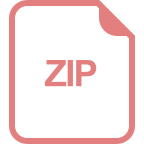
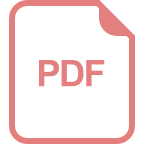
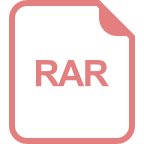
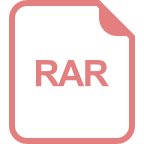
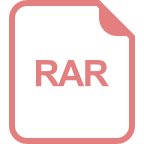
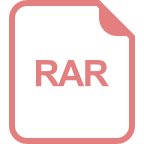
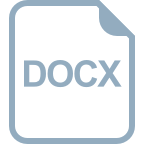
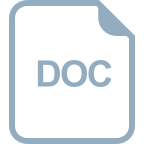
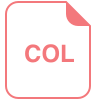