使用streamReader = new StreamReader(file.OpenReadStream())读取二进制文件到字节数组中
时间: 2024-09-14 08:12:07 浏览: 70
在.NET框架中,`StreamReader`主要是用于读取文本文件,如果需要读取二进制文件并将其转换为字节数组,通常会用到`FileStream`和`BinaryReader`结合。以下是步骤:
```csharp
using System.IO;
using System.IO.BinaryReader;
byte[] binaryData;
// 创建FileStream对象,它表示文件的二进制内容
FileStream fileStream = file.OpenReadStream();
try
{
// 创建BinaryReader对象,指定从FileStream读取
BinaryReader reader = new BinaryReader(fileStream);
// 一次性读取整个文件到字节数组
binaryData = reader.ReadBytes((int)fileStream.Length);
}
finally
{
// 关闭流,释放资源
if (reader != null)
reader.Close();
if (fileStream != null)
fileStream.Close();
}
// 现在binaryData数组包含了文件的二进制内容
```
相关问题
如何在c#webapi中接收前端发送的文件名,并读取文件中的二进制数据到字节数组中
在C# Web API中,接收前端发送的文件名并通过文件流读取其二进制数据到字节数组,你需要使用`FormData`对象以及`MultipartFormDataParser`来进行操作。以下是一个简单的示例:
首先,在前端发送文件时,你可以使用`FormData`对象:
```javascript
function uploadFile(file, fileName) {
var formData = new FormData();
formData.append("file", file, fileName);
$.ajax({
type: "POST",
url: "/api/upload",
data: formData,
cache: false,
contentType: false,
processData: false,
xhr: function () { // 创建自定义xhr对象
var xhr = new XMLHttpRequest();
xhr.upload.onprogress = function (e) {
if (e.lengthComputable) {
console.log(Math.round((e.loaded / e.total) * 100) + '% completed.');
}
};
return xhr;
},
success: function (response) {
console.log(response);
},
error: function (error) {
console.error(error);
}
});
}
```
在后端,你需要创建一个接受`multipart/form-data`请求的API:
```csharp
using Microsoft.AspNetCore.Http;
// ...
[HttpPost]
public async Task<IActionResult> Upload(IFormFile file)
{
if (file == null || file.Length == 0)
{
return BadRequest("No file selected.");
}
using (var streamReader = new StreamReader(file.OpenReadStream()))
{
var fileName = file.FileName;
var bytes = new byte[file.Length];
await streamReader.ReadAsync(bytes, 0, (int)file.Length);
// 这里可以进一步处理字节数据
// ...
}
return Ok($"File '{fileName}' has been received successfully.");
}
如何使用StreamReader配合Encoding.GetBytes来读取HEX文件?
在C#中,使用StreamReader和Encoding.GetBytes来读取HEX文件的基本步骤如下:
1. 打开文件:首先,创建一个FileStream或StreamReader实例,指定你要读取的HEX文件路径。例如:
```csharp
using (var file = new StreamReader("path_to_your_hex_file.hex"))
{
```
2. 逐行读取:使用StreamReader的ReadLine()方法按行读取文件内容:
```csharp
string line;
while ((line = file.ReadLine()) != null)
{
```
3. 解析十六进制:对于每一行,它通常由两个十六进制数表示一个字节,所以你需要提取并分割它们:
```csharp
string[] hexPair = line.Split(' ');
byte b;
if (hexPair.Length == 2)
{
b = Convert.ToByte(hexPair[0], 16); // 将第一个数字转换为字节
// 如果需要第二个数字(通常是校验位),可以类似处理
}
else
{
// 处理错误格式的行
}
```
4. 转换为字节数组:将单个字节添加到一个字节数组中,最后得到整个文件的字节序列:
```csharp
List<byte> byteArray = new List<byte>();
byteArray.Add(b); // 添加当前字节
// ...继续处理下一行,直到所有字节都被加入
byte[] finalByteArray = byteArray.ToArray();
// 现在finalByteArray包含了整个文件的内容
}
```
5. 关闭资源:记得在循环结束后关闭StreamReader:
```csharp
file.Close();
```
阅读全文
相关推荐
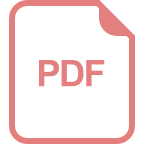
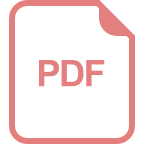
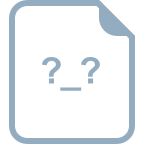
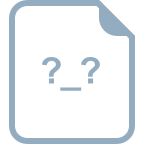
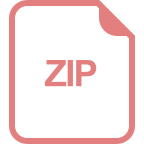
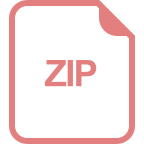
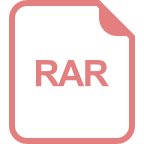
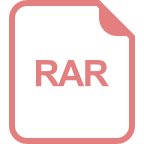
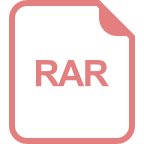
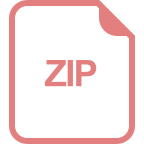
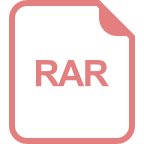
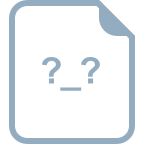
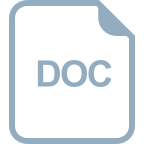
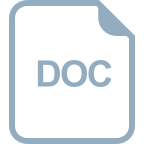
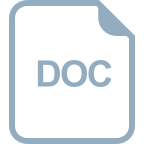

