c++多态代码实现
时间: 2024-04-19 22:20:40 浏览: 242
C++中多态的实现方式主要有以下几种:
1. 虚函数(Virtual Functions):通过在基类中声明虚函数,并在派生类中进行重写,实现多态性。当通过基类指针或引用调用虚函数时,会根据实际对象的类型来确定调用哪个函数。
示例代码如下:
```cpp
class Animal {
public:
virtual void sound() {
cout << "Animal makes a sound." << endl;
}
};
class Dog : public Animal {
public:
void sound() {
cout << "Dog barks." << endl;
}
};
class Cat : public Animal {
public:
void sound() {
cout << "Cat meows." << endl;
}
};
int main() {
Animal* animal1 = new Dog();
Animal* animal2 = new Cat();
animal1->sound(); // 输出:Dog barks.
animal2->sound(); // 输出:Cat meows.
delete animal1;
delete animal2;
return 0;
}
```
2. 纯虚函数(Pure Virtual Functions):在基类中声明纯虚函数,派生类必须实现该函数。纯虚函数使得基类成为抽象类,无法实例化,只能作为接口使用。
示例代码如下:
```cpp
class Shape {
public:
virtual void draw() = 0; // 纯虚函数
};
class Circle : public Shape {
public:
void draw() {
cout << "Drawing a circle." << endl;
}
};
class Rectangle : public Shape {
public:
void draw() {
cout << "Drawing a rectangle." << endl;
}
};
int main() {
Shape* shape1 = new Circle();
Shape* shape2 = new Rectangle();
shape1->draw(); // 输出:Drawing a circle.
shape2->draw(); // 输出:Drawing a rectangle.
delete shape1;
delete shape2;
return 0;
}
```
3. 虚析构函数(Virtual Destructors):当基类指针指向派生类对象时,如果基类的析构函数不是虚函数,那么只会调用基类的析构函数,而不会调用派生类的析构函数。为了正确释放派生类对象的资源,需要将基类的析构函数声明为虚函数。
示例代码如下:
```cpp
class Base {
public:
virtual ~Base() {
cout << "Base destructor." << endl;
}
};
class Derived : public Base {
public:
~Derived() {
cout << "Derived destructor." << endl;
}
};
int main() {
Base* base = new Derived();
delete base; // 输出:Derived destructor. Base destructor.
return 0;
}
```
阅读全文
相关推荐
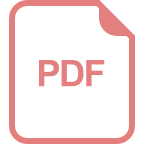
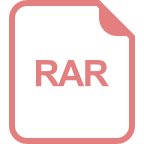
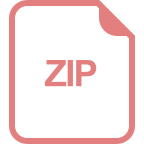















