五星红旗的Python代码
时间: 2024-10-02 11:00:18 浏览: 35
在Python中,可以使用PIL(Python Imaging Library)库来绘制五星红旗。这里是一个简单的例子,展示了如何用Python生成一个五角星形状:
```python
from PIL import Image, ImageDraw
# 创建一个白色的背景图像
img = Image.new('RGB', (100, 100), 'white')
draw = ImageDraw.Draw(img)
# 定义五角星的顶点坐标
vertices = [
[50, 90], # 第一个角
[80, 60],
[90, 30],
[80, 0],
[50, 30],
[20, 0],
[10, 30]
]
# 绘制五角星
for i in range(7):
draw.polygon([vertices[(i + j) % 7] for j in range(5)], fill='red') # 使用红色填充每个角
# 显示和保存图像
img.show()
img.save("flag.png")
```
这个代码会创建一个100x100像素的白色图片,并在其上绘制一个红色的五角星,代表中国的五星红旗。
相关问题
五星红旗python代码
五星红旗Python代码是一种可以用来绘制五星红旗的Python程序。使用Python语言编写的代码可以实现自动化绘制,大大提高了效率。以下是一个简单的五星红旗Python代码示例:
```
import turtle
# 设置画布大小
turtle.setup(600, 400)
# 绘制红色背景
turtle.penup()
turtle.goto(-300, 200)
turtle.pendown()
turtle.begin_fill()
turtle.fillcolor("red")
for i in range(2):
turtle.forward(600)
turtle.right(90)
turtle.forward(400)
turtle.right(90)
turtle.end_fill()
# 绘制大星星
turtle.penup()
turtle.goto(-180, 120)
turtle.pendown()
turtle.begin_fill()
turtle.fillcolor("yellow")
for i in range(5):
turtle.forward(80)
turtle.right(144)
turtle.end_fill()
# 绘制四个小星星
positions = [(10, 150), (-30, 100), (10, 70), (50, 100)]
for position in positions:
turtle.penup()
turtle.goto(position)
turtle.pendown()
turtle.begin_fill()
turtle.fillcolor("yellow")
for i in range(5):
turtle.forward(20)
turtle.right(144)
turtle.end_fill()
# 隐藏画笔
turtle.hideturtle()
# 等待用户关闭窗口
turtle.done()
```
以上代码可以使用Python的turtle库来绘制五星红旗,通过对不同位置、颜色和形状的绘制,最终得到一面完整的五星红旗。
绘画五星红旗python代码
当然,我可以给你介绍一下绘制五星红旗的Python代码。下面是一个简单的示例:
```python
import turtle
# 设置画布大小和背景颜色
turtle.setup(800, 600)
turtle.bgcolor("red")
# 绘制五角星的函数
def draw_star():
turtle.begin_fill()
for _ in range(5):
turtle.forward(100)
turtle.right(144)
turtle.end_fill()
# 绘制五星红旗
def draw_flag():
# 绘制大红旗
turtle.penup()
turtle.goto(-300, 200)
turtle.pendown()
turtle.color("yellow")
turtle.begin_fill()
for _ in range(2):
turtle.forward(600)
turtle.right(90)
turtle.forward(400)
turtle.right(90)
turtle.end_fill()
# 绘制大星星
turtle.penup()
turtle.goto(-220, 120)
turtle.pendown()
turtle.color("yellow")
draw_star()
# 绘制四颗小星星
positions = [(-120, 180), (-120, 60), (-40, 120), (-40, 0)]
for position in positions:
turtle.penup()
turtle.goto(position)
turtle.pendown()
turtle.color("yellow")
draw_star()
# 主函数
def main():
draw_flag()
turtle.hideturtle()
turtle.done()
if __name__ == '__main__':
main()
```
这段代码使用了Python的turtle库来绘制五星红旗。它首先设置了画布的大小和背景颜色,然后定义了一个绘制五角星的函数`draw_star()`。接着定义了一个绘制五星红旗的函数`draw_flag()`,其中使用了`draw_star()`函数来绘制五角星。最后,在主函数`main()`中调用`draw_flag()`函数来绘制五星红旗,并隐藏乌龟图标,保持绘图窗口的显示。
希望这段代码能够帮助到你!如果你有任何相关问题,请随时提问。
阅读全文
相关推荐
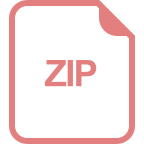
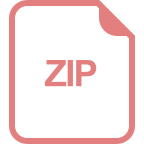













