python 利用继承和多态概念实现球的半径、颜色,性质、生成蓝球、水球、足球、铁球的球类
时间: 2024-06-24 13:02:21 浏览: 18
在Python中,我们可以使用继承和多态的概念来创建一个通用的"球"类,然后派生出具体的子类(如篮球、水球、足球和铁球)来表示不同类型的球,每个子类可以有自己的特性和行为。这里是一个简单的例子:
```python
# 基类球(Ball)
class Ball:
def __init__(self, radius, color):
self.radius = radius
self.color = color
# 定义抽象方法,用于展示多态性
def bounce(self):
raise NotImplementedError("Subclasses must implement this method.")
# 简单的描述方法
def describe(self):
return f"{self.color} ball with a radius of {self.radius}."
# 子类篮球(Basketball),继承自Ball
class Basketball(Ball):
def bounce(self):
return f"{self.color} basketball bounces!"
# 子类水球(WaterBall)
class WaterBall(Ball):
def bounce(self):
return f"{self.color} water ball gently floats."
# 其他子类...
class Football(Ball):
def bounce(self):
return f"{self.color} football kicks off with a thud."
class IronBall(Ball):
def bounce(self):
return f"{self.color} iron ball makes a metallic sound when dropped."
# 使用示例
blue_ball = Basketball(7, "blue")
print(blue_ball.describe())
print(blue_ball.bounce()) # 输出: blue ball with a radius of 7.
water_ball = WaterBall(6, "clear")
print(water_ball.describe())
print(water_ball.bounce()) # 输出: clear water ball gently floats.
```
在这个例子中,`Ball`是父类,`Basketball`, `WaterBall`, `Football`, 和 `IronBall`是子类。`bounce()`方法被声明为抽象方法,子类需要实现自己的版本以体现多态性。每个子类可以有自己的颜色和可能的行为。
相关推荐
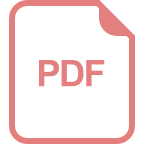
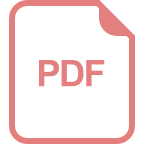
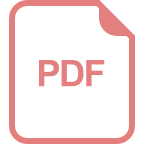














