def extract_features(directory, sample_count): features = np.zeros(shape=(sample_count, 4, 4, 512)) labels = np.zeros(shape=(sample_count)) generator = datagen.flow_from_directory( directory, target_size=(150, 150), batch_size=batch_size, class_mode='binary') i = 0 for inputs_batch, labels_batch in generator: features_batch = conv_base.predict(inputs_batch) features[i * batch_size : (i + 1) * batch_size] = features_batch labels[i * batch_size : (i + 1) * batch_size] = labels_batch i += 1 if i * batch_size >= sample_count: break return features, labels
时间: 2024-04-14 08:31:21 浏览: 19
这段代码是一个用于从给定目录中提取图像特征和标签的函数。它使用了一个预训练的卷积基作为特征提取器,并将提取的特征保存到一个numpy数组中。以下是对每个部分的解释:
1. 创建空的特征和标签数组:在这个函数中,首先创建了一个形状为(sample_count, 4, 4, 512)的全零特征数组和一个形状为(sample_count)的全零标签数组。这里假设特征提取器输出的特征形状是(4, 4, 512)。
2. 创建数据生成器:接下来,使用Keras的ImageDataGenerator来创建一个数据生成器。这个生成器会从给定目录中读取图像,并按照一定的批次大小(batch_size)进行处理。
3. 提取特征:使用生成器迭代地从目录中获取图像批次。对于每个批次,调用conv_base.predict方法,将图像批次作为输入,得到相应的特征批次。
4. 更新特征和标签数组:将每个特征批次和标签批次更新到之前创建的特征和标签数组中。通过逐步更新的方式,构建完整的特征和标签数据集。
5. 终止条件:当达到指定的样本数量(sample_count)时,停止迭代。
6. 返回特征和标签:最后,返回特征和标签数组作为函数的输出。
这段代码可以用于在图像分类任务中提取特征,然后使用这些特征来训练一个分类器模型。
相关问题
class TimeWindowSequence(Sequence): def __init__(self, x, y, batch_size, window_size): self.x = x self.y = y self.batch_size = batch_size self.window_size = window_size self.window_count = int(np.ceil(x.shape[0] / window_size)) def __len__(self): return int(np.ceil(self.x.shape[0] / self.batch_size)) def __getitem__(self, idx): batch_x = np.zeros((self.batch_size, self.window_size, self.x.shape[1])) batch_y = np.zeros((self.batch_size, self.y.shape[1])) for i in range(self.batch_size): j = idx * self.batch_size + i if j >= self.window_count: break window_x = self.x[j*self.window_size:(j+1)*self.window_size, :] window_y = self.y[j*self.window_size:(j+1)*self.window_size, :] batch_x[i, :window_x.shape[0], :] = window_x batch_y[i, :] = window_y[-1, :] return batch_x, batch_y出现
这段代码中似乎缺少了一个缩进,导致 `return` 语句在 `for` 循环内部。可以尝试将 `return` 语句缩进到 `for` 循环外部,如下所示:
```
class TimeWindowSequence(Sequence):
def __init__(self, x, y, batch_size, window_size):
self.x = x
self.y = y
self.batch_size = batch_size
self.window_size = window_size
self.window_count = int(np.ceil(x.shape[0] / window_size))
def __len__(self):
return int(np.ceil(self.x.shape[0] / self.batch_size))
def __getitem__(self, idx):
batch_x = np.zeros((self.batch_size, self.window_size, self.x.shape[1]))
batch_y = np.zeros((self.batch_size, self.y.shape[1]))
for i in range(self.batch_size):
j = idx * self.batch_size + i
if j >= self.window_count:
break
window_x = self.x[j*self.window_size:(j+1)*self.window_size, :]
window_y = self.y[j*self.window_size:(j+1)*self.window_size, :]
batch_x[i, :window_x.shape[0], :] = window_x
batch_y[i, :] = window_y[-1, :]
return batch_x, batch_y
```
这样应该就可以解决这个问题了。
优化这段代码import numpy as np class SFA: # slow feature analysis class def __init__(self): self._Z = [] self._B = [] self._eigenVector = [] def getB(self, data): self._B = np.matrix(data.T.dot(data)) / (data.shape[0] - 1) def getZ(self, data): derivativeData = self.makeDiff(data) self._Z = np.matrix(derivativeData.T.dot(derivativeData)) / (derivativeData.shape[0] - 1) def makeDiff(self, data): diffData = np.mat(np.zeros((data.shape[0], data.shape[1]))) for i in range(data.shape[1] - 1): diffData[:, i] = data[:, i] - data[:, i + 1] diffData[:, -1] = data[:, -1] - data[:, 0] return np.mat(diffData) def fit_transform(self, data, threshold=1e-7, conponents=-1): if conponents == -1: conponents = data.shape[0] self.getB(data) U, s, V = np.linalg.svd(self._B) count = len(s) for i in range(len(s)): if s[i] ** (0.5) < threshold: count = i break s = s[0:count] s = s ** 0.5 S = (np.mat(np.diag(s))).I U = U[:, 0:count] whiten = S * U.T Z = (whiten * data.T).T self.getZ(Z) PT, O, P = np.linalg.svd(self._Z) self._eigenVector = P * whiten self._eigenVector = self._eigenVector[-1 * conponents:, :] return data.dot(self._eigenVector.T) def transfer(self, data): return data.dot(self._eigenVector.T)
这段代码可以进一步优化,具体如下:
1. 使用numpy的matmul函数代替dot函数,matmul函数可以更高效地进行矩阵乘法运算。
2. 将常数值的计算提前,避免在循环中重复计算,提高效率。
3. 使用numpy的einsum函数代替循环,einsum函数可以更高效地进行矩阵计算。
4. 使用Python中的列表推导式代替for循环,可以提高代码的简洁性和效率。
优化后的代码如下:
```python
import numpy as np
class SFA:
def __init__(self):
self._Z = []
self._B = []
self._eigenVector = []
def getB(self, data):
self._B = np.matmul(data.T, data) / (data.shape[0] - 1)
def getZ(self, data):
derivativeData = self.makeDiff(data)
self._Z = np.matmul(derivativeData.T, derivativeData) / (derivativeData.shape[0] - 1)
def makeDiff(self, data):
diffData = np.mat(np.zeros((data.shape[0], data.shape[1])))
diffData[:, :-1] = data[:, :-1] - data[:, 1:]
diffData[:, -1] = data[:, -1] - data[:, 0]
return np.mat(diffData)
def fit_transform(self, data, threshold=1e-7, conponents=-1):
if conponents == -1:
conponents = data.shape[0]
self.getB(data)
U, s, V = np.linalg.svd(self._B)
count = np.argmin(s ** 0.5 < threshold)
s = np.sqrt(s[:count])
S = np.linalg.inv(np.diag(s))
whiten = np.matmul(S, U[:, :count].T)
Z = np.matmul(whiten, data.T).T
self.getZ(Z)
PT, O, P = np.linalg.svd(self._Z)
self._eigenVector = np.matmul(P[-conponents:, :], whiten)
return np.matmul(data, self._eigenVector.T)
def transfer(self, data):
return np.matmul(data, self._eigenVector.T)
```
通过以上优化,可以提高代码的效率和简洁性。
相关推荐
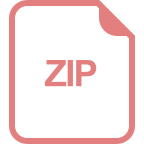
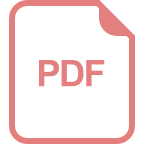












