<?php // 连接数据库 $conn = mysqli_connect("localhost", "tms", "123456", "nut"); // 检查连接是否成功 if (!$conn) { die("数据库连接失败: " . mysqli_connect_error()); }echo "111"; // 处理表单提交 if ($_SERVER["REQUEST_METHOD"] == "POST") { // 获取表单数据 $aoiStep = $_POST['aoi_step']; $defectType = $_POST['defect_type']; $layerCode = $_POST['layer_code']; $type = $_POST['type']; $dpet = $_POST['dpet']; $subcode = $_POST['subcode']; $codeDescription = $_POST['code_description']; $determinationRule = $_POST['determination_rule']; $imagePaths = []; // 存储图片路径的数组 // 处理上传的图片 for ($i = 1; $i <= 5; $i++) { $imageField = "image" . $i; $targetDir = "D:/phpstudy_pro/WWW/192.168.1.16/images"; // 设置上传目录的路径 $fileName = uniqid() . '_' . $_FILES[$imageField]["name"]; // 生成唯一文件名 $targetFile = $targetDir . '/' . basename($fileName); // 将反斜杠替换为正斜杠 $targetFile = str_replace('\', '/', $targetFile); if (isset($_FILES[$imageField]) && $_FILES[$imageField]["error"] == UPLOAD_ERR_OK && move_uploaded_file($_FILES[$imageField]["tmp_name"], $targetFile)) { $imagePath = $targetFile; } else { $imagePath = ""; } $imagePaths[] = $imagePath; } // 其他图片的处理代码,类似上面的处理方式 $stmt = $conn->prepare("INSERT INTO tms (aoi_step, defect_type, layer_code, type, dpet, subcode, code_description, image1_path, image2_path, image3_path, image4_path, image5_path, determination_rule) VALUES (?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?)"); if (!$stmt) { die("预处理失败: " . $conn->error); } $stmt->bind_param("sssssssssssss", $aoiStep, $defectType, $layerCode, $type, $dpet, $subcode, $codeDescription, $imagePaths[0], $imagePaths[1], $imagePaths[2], $imagePaths[3], $imagePaths[4], $determinationRule); if ($stmt->execute()) { echo "数据插入成功"; } else { echo "数据插入失败: " . $stmt->error; } // 关闭数据库连接 mysqli_close($conn); } ?>在这个代码中,当我有多行数据时,只会上传最后一条,我需要怎么修改,才能将所有编辑的数据都进行上传
时间: 2023-08-31 11:08:13 浏览: 114
在你的代码中,你只处理了一个表单数据,并且只上传了最后一条数据。如果你想上传所有编辑的数据,你需要做以下修改:
1. 在 HTML 表单中,将表单元素的名称改为数组形式,以便能够接收多个值。例如,将 `aoi_step` 改为 `aoi_step[]`,将 `defect_type` 改为 `defect_type[]`,以此类推。这样,当你选择多个选项时,它们会以数组的形式传递给服务器。
2. 在处理表单提交的代码中,使用循环来处理每个表单元素的值。你可以使用 `foreach` 循环来遍历每个表单元素的数组,并将它们插入到数据库中。
下面是修改后的代码示例:
```php
// 连接数据库
$conn = mysqli_connect("localhost", "tms", "123456", "nut");
// 检查连接是否成功
if (!$conn) {
die("数据库连接失败: " . mysqli_connect_error());
}
// 处理表单提交
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// 获取表单数据
$aoiSteps = $_POST['aoi_step'];
$defectTypes = $_POST['defect_type'];
$layerCodes = $_POST['layer_code'];
$types = $_POST['type'];
$dpets = $_POST['dpet'];
$subcodes = $_POST['subcode'];
$codeDescriptions = $_POST['code_description'];
$determinationRules = $_POST['determination_rule'];
$imagePaths = []; // 存储图片路径的数组
// 处理上传的图片
for ($i = 1; $i <= 5; $i++) {
$imageField = "image" . $i;
$targetDir = "D:/phpstudy_pro/WWW/192.168.1.16/images"; // 设置上传目录的路径
$fileName = uniqid() . '_' . $_FILES[$imageField]["name"]; // 生成唯一文件名
$targetFile = $targetDir . '/' . basename($fileName);
// 将反斜杠替换为正斜杠
$targetFile = str_replace('\\', '/', $targetFile);
if (isset($_FILES[$imageField]) && $_FILES[$imageField]["error"] == UPLOAD_ERR_OK && move_uploaded_file($_FILES[$imageField]["tmp_name"], $targetFile)) {
$imagePath = $targetFile;
} else {
$imagePath = "";
}
$imagePaths[] = $imagePath;
}
// 插入数据
$stmt = $conn->prepare("INSERT INTO tms (aoi_step, defect_type, layer_code, type, dpet, subcode, code_description, image1_path, image2_path, image3_path, image4_path, image5_path, determination_rule) VALUES (?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?)");
if (!$stmt) {
die("预处理失败: " . $conn->error);
}
foreach ($aoiSteps as $key => $aoiStep) {
// 绑定参数
$stmt->bind_param("sssssssssssss", $aoiStep, $defectTypes[$key], $layerCodes[$key], $types[$key], $dpets[$key], $subcodes[$key], $codeDescriptions[$key], $imagePaths[0], $imagePaths[1], $imagePaths[2], $imagePaths[3], $imagePaths[4], $determinationRules[$key]);
if ($stmt->execute()) {
echo "数据插入成功";
} else {
echo "数据插入失败: " . $stmt->error;
}
}
// 关闭数据库连接
mysqli_close($conn);
}
```
在上述代码中,我们使用了 `foreach` 循环来遍历每个表单元素的数组,并将它们绑定到 SQL 语句中。这样,每个编辑的数据都会被插入到数据库中。请确保你的 HTML 表单中的每个表单元素都以数组的形式命名,以便能够接收多个值。
阅读全文
相关推荐
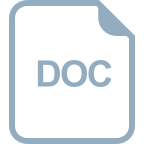
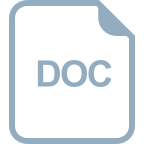
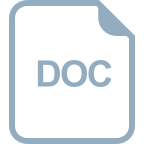



<?php // 连接数据库 $conn = new mysqli("localhost", "root", "123456", "wyya"); // 检查连接是否成功 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 查询五个歌单的表 $tables = array(); $result = mysqli_query($conn, "SHOW TABLES LIKE '%_list'"); if ($result->num_rows > 0) { while ($row = mysqli_fetch_array($result)) { $tables[] = $row[0]; } } // 获取选中的歌单表 $tableName = isset($_GET["table"]) ? $_GET["table"] : ""; $data = array(); if (!empty($tableName)) { $result = mysqli_query($conn, "SELECT * FROM $tableName"); if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { $data[] = $row; } } } ?> <!DOCTYPE html> <html> <head> <title>网易云音乐歌单</title> <style> table { border-collapse: collapse; width: 100%; } th, td { text-align: left; padding: 8px; } tr:nth-child(even) { background-color: #f2f2f2; } th { background-color: #4CAF50; color: white; } </style> </head> <body> 网易云音乐歌单 华语 欧美 日语 韩语 粤语 <?php if (!empty($tableName)) { ?> <?php $columns = mysqli_query($conn, "SHOW COLUMNS FROM $tableName"); if ($columns->num_rows > 0) { while ($column = mysqli_fetch_array($columns)) { ?> <?php echo $column["Field"]; ?> <?php } } ?> <?php foreach ($data as $row) { ?> <?php foreach ($row as $value) { ?> <?php echo $value; ?> <?php } ?> <?php } ?> <?php } ?> </body> </html> <?php // 关闭连接 $conn->close(); ?>修改代码,将华语,欧美,日语,韩语,粤语放入一个命名为分类的菜单中,实现点击菜单后点击华语,欧美,日语,韩语,粤语再跳转


检查这段代码的错误并修改实现与book.php的跳转<?php header("Location: book.php"); //连接数据库 $servername = "127.0.0.12"; $username = "roots"; $password = "1234root"; $dbname = "zkw"; $conn = mysqli_connect($servername, $username, $password, $dbname); if (!$conn) { die("连接失败: " . mysqli_connect_error()); } //查询图书 $id = $_GET["id"]; $sql = "SELECT * FROM books WHERE id=$id"; $result = mysqli_query($conn, $sql); $row = mysqli_fetch_assoc($result); //检查用户是否登录 session_start(); if (!isset($_SESSION["username"])) { $bookshelf_text = "登录后可以添加到书架"; } else { $username = $_SESSION["username"]; $sql = "SELECT * FROM bookshelf WHERE username='$username' AND book_id=$id"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { $bookshelf_text = "从书架中移除"; } else { $bookshelf_text = "添加到书架"; } } mysqli_close($conn); ?> <!DOCTYPE html> <html> <head> <title><?php echo $row["name"]; ?></title> </head> <body> <?php echo $row["name"]; ?> <?php echo $row["description"]; ?> <?php echo $bookshelf_text; ?> </body> </html>







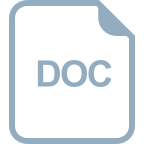
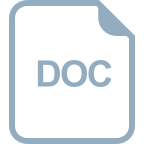