在不适用main函数的情况下,用Java实现基于socketTCP协议的*.csv文件的下载
时间: 2024-10-26 08:16:23 浏览: 23
在Java中,如果你想要实现一个TCP服务器,用于从远程服务器下载CSV文件,并且不使用`main`函数直接启动服务,你可以创建一个`ServerSocket`监听端口并处理客户端连接。以下是一个简化的示例,展示如何通过Socket通信接收文件数据:
```java
import java.io.*;
import java.net.*;
public class CSVDownloaderServer {
private static final int PORT = 8000; // 设置监听的端口号
public static void start() throws IOException {
ServerSocket serverSocket = new ServerSocket(PORT);
System.out.println("CSV download server started on port " + PORT);
try (Socket clientSocket = serverSocket.accept()) {
InputStream in = clientSocket.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
String line;
while ((line = reader.readLine()) != null) { // 接收CSV数据行
if (line.startsWith("Content-Disposition")) { // 检查头信息是否表示开始下载
// 根据HTTP header解析文件名和路径
String fileName = extractFileName(line);
File outputFile = createTempFile(fileName);
OutputStream out = new FileOutputStream(outputFile); // 创建临时文件保存接收到的数据
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
out.close(); // 关闭输出流
System.out.println("Downloaded file to: " + outputFile.getAbsolutePath());
break; // 文件已接收完毕,跳出循环
}
}
} finally {
serverSocket.close();
}
}
private static String extractFileName(String contentDispositionLine) {
// 使用正则表达式提取文件名和路径,这里仅作简单示例,实际使用需考虑复杂情况
return contentDispositionLine.substring(contentDispositionLine.indexOf("filename=") + "filename=".length()).trim();
}
private static File createTempFile(String fileName) throws IOException {
File tempDir = Files.createTempDirectory("download").toFile(); // 创建临时目录
return new File(tempDir, fileName);
}
public static void main(String[] args) {
try {
CSVDownloaderServer.start();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这个例子中,你需要在主方法中启动服务:
```java
public static void main(String[] args) {
CSVDownloaderServer.main(null); // 调用start方法而不是直接运行这个类
}
```
这个程序只是一个基本框架,真实的生产环境部署需要考虑异常处理、线程安全以及更复杂的网络协议解析。另外,这个示例假设服务器发送的是完整的HTTP响应头,包括文件名称,实际应用中可能需要使用专门的库(如HttpURLConnection或OkHttp)来更好地处理请求。
阅读全文
相关推荐
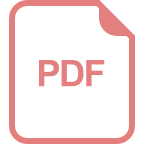
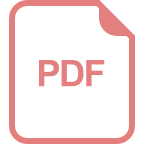
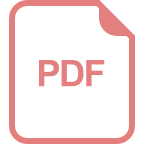

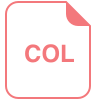
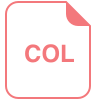
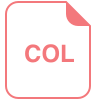
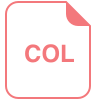
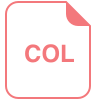
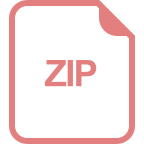
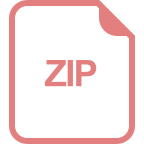
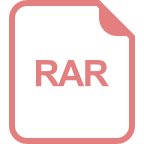
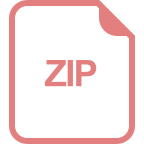
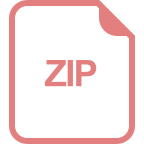
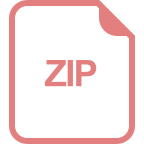