php与html交互实现单个文件上传。上传文件后跳转为php代码
时间: 2024-10-24 14:13:22 浏览: 29
在PHP和HTML结合的情况下,实现单个文件上传通常涉及到前端表单提交和后端处理两部分。首先,我们创建一个HTML表单让用户选择文件:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>File Upload</title>
</head>
<body>
<form action="upload.php" method="post" enctype="multipart/form-data">
<input type="file" name="fileToUpload" id="fileToUpload">
<input type="submit" value="Upload File">
</form>
</body>
</html>
```
在这个`index.html`文件中,`action`属性指向`upload.php`,`enctype`设置为`multipart/form-data`是因为我们需要处理二进制数据,如文件。
接下来,是`upload.php`文件,它接收并处理来自前端的文件上传请求:
```php
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$target_dir = "uploads/";
$target_file = $target_dir . basename($_FILES["fileToUpload"]["name"]);
$uploadOk = 1;
// 检查文件是否已经存在
if (file_exists($target_file)) {
echo "Sorry, file already exists.";
$uploadOk = 0;
}
// 检查文件大小
if ($_FILES["fileToUpload"]["size"] > 500000) { // 这里是一个例子,你可以调整为需要的最大大小
echo "Sorry, your file is too large.";
$uploadOk = 0;
}
// 允许上传的文件类型
$allowed_types = array("jpg", "jpeg", "png", "pdf"); // 添加你需要支持的文件类型
$file_type = strtolower(pathinfo($target_file,PATHINFO_EXTENSION));
if (!in_array($file_type, $allowed_types)) {
echo "Sorry, only JPG, JPEG, PNG & PDF files are allowed.";
$uploadOk = 0;
}
if ($uploadOk == 0) {
echo "Sorry, your file was not uploaded.";
} else {
if (move_uploaded_file($_FILES["fileToUpload"]["tmp_name"], $target_file)) {
echo "The file ". htmlspecialchars( basename( $_FILES["fileToUpload"]["name"])). " has been uploaded.";
// 如果你想要跳转到其他PHP页面,可以在这里添加header或redirect
header("Location: success.php");
} else {
echo "Sorry, there was an error uploading your file.";
}
}
}
?>
```
在这个`upload.php`中,如果文件上传成功,会将文件移动到服务器的`uploads/`目录,并通过`header()`函数发送用户到`success.php`页面。如果遇到任何错误,会显示相应的错误消息。
阅读全文
相关推荐













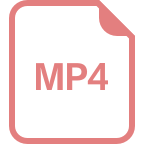