C#怎么用接口定义一个集合
时间: 2024-09-21 13:01:55 浏览: 34
在C#中,接口用于定义一组规范,让实现了该接口的类具备特定的功能。要定义一个接口用于表示集合,你可以按照以下步骤操作:
1. **创建接口**:
```csharp
public interface IMyCollection<T>
{
// 定义集合的基本属性和方法
int Count { get; }
void Add(T item);
bool Remove(T item);
T this[int index] { get; set; }
// 可选的泛型方法,如搜索、排序等
T FindBySomeProperty(string propertyValue);
List<T> SortedList();
}
```
这里,`IMyCollection<T>`是一个模板接口,T代表了集合中的元素类型。
2. **实现接口**:
任何想要作为“我的集合”的类都需实现这个接口,例如:
```csharp
public class MyArrayList : IMyCollection<int>
{
private List<int> items;
public int Count => items.Count;
public void Add(int item) => items.Add(item);
public bool Remove(int item) => items.Remove(item);
public int this[int index] => items[index];
public int FindBySomeProperty(string propertyValue) { /* 实现查找逻辑 */ }
public List<int> SortedList() => items.OrderBy(x => x).ToList(); // 使用 LINQ 排序
}
```
3. **使用接口**:
通过接口名来引用实现了接口的类,可以保证它们共享相同的接口规范:
```csharp
IMyCollection<int> myCollection = new MyArrayList();
myCollection.Add(10); // 不关心实际是List还是其他实现
```
阅读全文
相关推荐
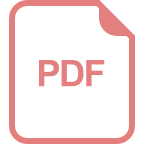
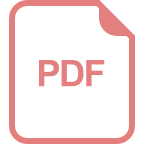
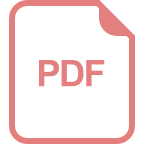
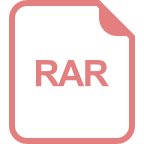
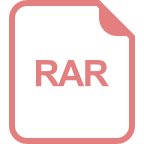
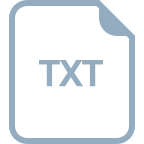
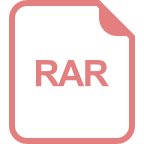
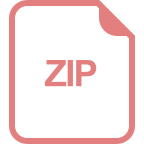
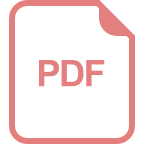
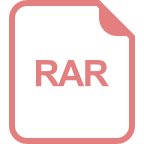
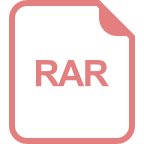
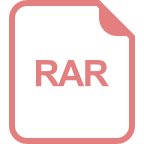
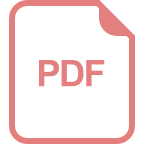
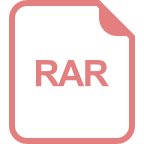
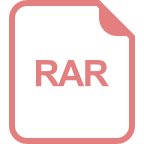
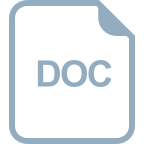
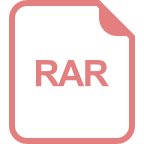
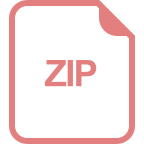
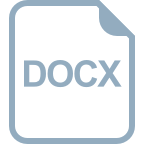