详细代码智能简历解析系统Java
时间: 2023-10-11 21:11:31 浏览: 470
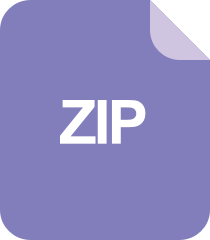
Java实现的智能灯控制系统源代码,智能开关灯系统源代码,大学实训
Java代码实现智能简历解析系统,需要用到自然语言处理(NLP)和机器学习技术,主要步骤如下:
1. 数据采集和预处理:从各种渠道收集大量的简历数据,并进行数据清洗和预处理,去除无用信息,提取关键信息,例如姓名、联系方式、教育经历、工作经历等。
2. 特征提取:根据预处理后的数据,提取出重要的特征,例如学历、专业、工作年限、技能等,并将其转化为数值型特征,以便于机器学习算法处理。
3. 模型训练和选择:选择合适的机器学习算法,例如决策树、随机森林、逻辑回归等,根据提取的特征对模型进行训练,以实现从简历中提取关键信息的目的。
4. 简历解析:使用训练好的模型对新的简历进行解析,提取出相应的信息,例如姓名、联系方式、教育经历、工作经历等,并将其存储到数据库中。
5. 智能推荐:根据解析后的信息,结合职位需求,进行匹配和推荐合适的候选人,以提高招聘效率和准确性。
下面是一个简单的Java实现示例:
```java
// 数据预处理
public class ResumePreprocessor {
public static Resume preprocess(String resumeText) {
// 去除非文字信息,例如图片、表格等
String text = removeNonTextInformation(resumeText);
// 提取关键信息,例如姓名、联系方式、教育经历、工作经历等
String name = extractName(text);
String email = extractEmail(text);
List<Education> educationList = extractEducation(text);
List<Experience> experienceList = extractExperience(text);
// 构建简历对象
Resume resume = new Resume(name, email, educationList, experienceList);
return resume;
}
}
// 特征提取
public class ResumeFeatureExtractor {
public static Map<String, Double> extractFeatures(Resume resume) {
Map<String, Double> featureMap = new HashMap<>();
// 提取学历、专业、工作年限、技能等特征,并转化为数值型特征
double educationLevel = extractEducationLevel(resume);
double workYears = extractWorkYears(resume);
double skillLevel = extractSkillLevel(resume);
featureMap.put("educationLevel", educationLevel);
featureMap.put("workYears", workYears);
featureMap.put("skillLevel", skillLevel);
return featureMap;
}
}
// 模型训练和选择
public class ResumeClassifier {
private static final String MODEL_FILE = "resume_classifier.model";
private static final List<String> FEATURE_LIST = Arrays.asList("educationLevel", "workYears", "skillLevel");
private DecisionTreeModel model;
public ResumeClassifier() {
// 加载训练好的模型
model = DecisionTreeModel.load(MODEL_FILE);
}
public boolean classify(Resume resume) {
// 提取特征
Map<String, Double> featureMap = ResumeFeatureExtractor.extractFeatures(resume);
List<LabeledPoint> data = new ArrayList<>();
// 将特征转化为LabeledPoint类型,以便于模型预测
LabeledPoint labeledPoint = new LabeledPoint(1.0, Vectors.dense(
featureMap.get("educationLevel"),
featureMap.get("workYears"),
featureMap.get("skillLevel")
));
data.add(labeledPoint);
Dataset<Row> testData = SparkUtils.spark().createDataFrame(data, LabeledPoint.class).toDF();
// 使用模型进行预测
double prediction = model.predict(testData.head().features());
return prediction == 1.0;
}
}
// 简历解析
public class ResumeParser {
private static final String RESUME_FOLDER = "resumes";
private static final String DATABASE_URL = "jdbc:mysql://localhost:3306/resume_db";
private static final String USERNAME = "root";
private static final String PASSWORD = "password";
private static final String DRIVER_CLASS = "com.mysql.jdbc.Driver";
private static final String INSERT_SQL = "INSERT INTO resume (name, email, education, experience) VALUES (?, ?, ?, ?)";
public static void main(String[] args) {
// 获取简历文件列表
List<File> resumeFiles = getResumeFiles(RESUME_FOLDER);
// 初始化数据库连接
try (Connection conn = DriverManager.getConnection(DATABASE_URL, USERNAME, PASSWORD)) {
// 解析每个简历文件,并将其存储到数据库中
for (File resumeFile : resumeFiles) {
String resumeText = FileUtils.readFileToString(resumeFile, StandardCharsets.UTF_8);
Resume resume = ResumePreprocessor.preprocess(resumeText);
if (resume != null) {
boolean isQualified = new ResumeClassifier().classify(resume);
if (isQualified) {
PreparedStatement stmt = conn.prepareStatement(INSERT_SQL);
stmt.setString(1, resume.getName());
stmt.setString(2, resume.getEmail());
stmt.setString(3, gson.toJson(resume.getEducationList()));
stmt.setString(4, gson.toJson(resume.getExperienceList()));
stmt.executeUpdate();
}
}
}
} catch (SQLException | IOException e) {
e.printStackTrace();
}
}
}
// 智能推荐
public class CandidateMatcher {
private static final String DATABASE_URL = "jdbc:mysql://localhost:3306/resume_db";
private static final String USERNAME = "root";
private static final String PASSWORD = "password";
private static final String DRIVER_CLASS = "com.mysql.jdbc.Driver";
private static final String SELECT_SQL = "SELECT * FROM resume WHERE education LIKE ? AND experience LIKE ?";
public static List<Resume> findMatches(String education, String experience) {
List<Resume> matches = new ArrayList<>();
// 初始化数据库连接
try (Connection conn = DriverManager.getConnection(DATABASE_URL, USERNAME, PASSWORD)) {
PreparedStatement stmt = conn.prepareStatement(SELECT_SQL);
stmt.setString(1, "%" + education + "%");
stmt.setString(2, "%" + experience + "%");
ResultSet rs = stmt.executeQuery();
// 将匹配的简历转化为对象
while (rs.next()) {
String name = rs.getString("name");
String email = rs.getString("email");
String educationJson = rs.getString("education");
String experienceJson = rs.getString("experience");
List<Education> educationList = gson.fromJson(educationJson, new TypeToken<List<Education>>() {}.getType());
List<Experience> experienceList = gson.fromJson(experienceJson, new TypeToken<List<Experience>>() {}.getType());
Resume resume = new Resume(name, email, educationList, experienceList);
matches.add(resume);
}
} catch (SQLException e) {
e.printStackTrace();
}
return matches;
}
}
```
以上代码仅供参考,实际实现需要根据具体需求进行调整和优化。
阅读全文
相关推荐
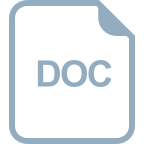
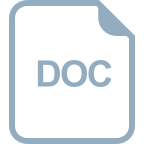
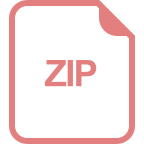
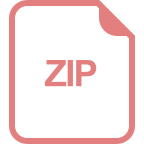
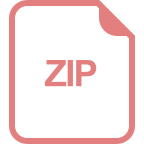
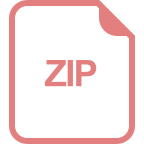
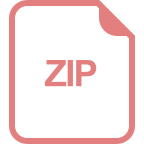
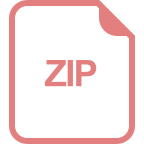
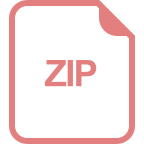
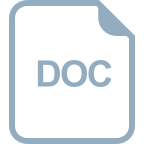
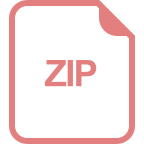
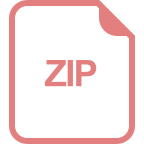
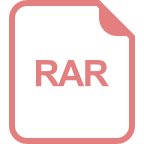
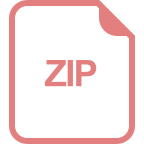
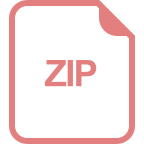
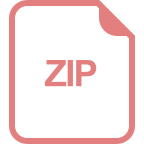
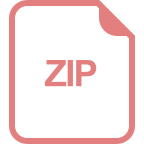
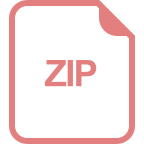